
Applying Clean Code Principles in Full Stack Development: A Comprehensive Guide
Apr 30, 2024 7 Min Read 412 Views
(Last Updated)
In the realm of full stack development, adhering to clean code principles is not just a practice, but a necessity for ensuring maintainability, readability, and effective collaboration.
With the burgeoning complexity of projects, clean code acts as the linchpin that holds the intricate machinery of coding standards, ranging from comments for clarity to employing polymorphism for cleaner logic.
It champions the cause of simplicity in code, embodying the essence of easy-to-read, understand, and modify constructs while laying down a foundation for consistent best practices.
This guide aims to elevate your coding paradigm by integrating essential clean code metrics, from polymorphism to the paramount importance of maintainability, ensuring your full stack development endeavors are built on a bedrock of high-quality, maintainable code.
Table of contents
- Understanding Clean Code
- Key Principles of Clean Code
- 1) Emphasize Simplicity and Readability
- 2) Adhere to DRY and Avoid Complexity
- 3) Consistent Practices and Error Management
- Best Practices for Writing Clean Code
- 1) Meaningful Naming and Function Management
- 2) Strategic Use of Comments and Documentation
- 3) Consistency and Code Structure
- Common Pitfalls to Avoid
- 1) Insufficient Planning and Design
- 2) Ignoring Code Readability and Maintainability
- 3) Not Handling Errors and Exceptions Properly
- 4) Overlooking Security Vulnerabilities
- 5) Poor Version Control Practices
- Tools and Techniques for Maintaining Clean Code
- Identify and Implement Effective Tools and Techniques
- Emphasize Code Quality and Maintenance
- Utilize Advanced Development Practices
- Leveraging Version Control for Collaboration
- Key Concepts of Version Control
- Collaborative Tools and Practices
- Best Practices for Collaborative Development Using Git
- Error Handling and Code Robustness
- 1) Implementing Effective Error Handling Strategies
- 2) Comprehensive Logging and Exception Management
- 3) Continuous Improvement of Error Handling Practices
- Implementing Refactoring and Testing
- Concluding Thoughts...
- FAQs
- What are the fundamental principles of clean code?
- Is the "Clean Code" book suitable for beginner programmers?
- How long is the "Clean Code" book?
Understanding Clean Code
Clean code is fundamental in full stack development, serving as the backbone for creating maintainable, error-free, and fast applications. At its core, clean code is characterized by simplicity and readability, making it easy for any developer to understand and modify. This simplicity is crucial, as it directly correlates with reduced development times and fewer bugs.
- Essential Attributes of Clean Code:
- Readability: Just like well-written prose, every line of code should be clear and purposeful.
- Maintainability: The code should be written in a way that others can easily pick up and expand or modify without the need for extensive rework.
- Functionality: It must function correctly and efficiently under all expected conditions.
- Best Practices:
- Consistency in Coding Standards: Using consistent naming conventions and formatting styles helps maintain uniformity.
- Refactoring and Testing: Regular updates and testing ensure the code stays robust and performant.
- Tools for Clean Code:
- SonarLint, SonarQube, and SonarCloud: These tools support continuous inspection of code quality, helping developers detect and fix issues before they become problematic.
By adhering to these principles and utilizing the right tools, developers can enhance collaboration and increase the overall quality of their software, making each project a long-term asset.
Would you like to master Full Stack Development and build an impressive portfolio? Then GUVI’s Full Stack Development Bootcamp is the perfect choice for you, taught by industry experts, this boot camp equips you with everything you need to know along with extensive placement assistance!
Key Principles of Clean Code
Let us now discuss at length what the key principles to writing clean code are and how you can implement them:
1) Emphasize Simplicity and Readability
Clean code is fundamentally about simplicity and readability. This means writing code that any developer can understand quickly, not just those who wrote it.
To achieve this, use meaningful and descriptive names for variables, functions, and classes that reflect their purpose and behavior.
Keep your functions and methods short, focusing each on a single task. This not only makes the code more digestible but also enhances its maintainability.
Find Out How much DSA for Full Stack Development Is Required?
2) Adhere to DRY and Avoid Complexity
The DRY (Don’t Repeat Yourself) principle is crucial in avoiding redundancy, which can lead to maintenance nightmares.
Ensure that each piece of knowledge or logic in your codebase has a single, unambiguous representation. Additionally, apply the YAGNI (You Aren’t Gonna Need It) principle to prevent over-engineering by not implementing features until they are necessary.
This approach helps keep the codebase clean and efficient, focusing on what is needed at the moment.
3) Consistent Practices and Error Management
Maintaining consistency across the codebase is key to clean code. This includes consistent naming conventions, coding styles, and formatting.
Such consistency makes the code easier to read and less error-prone. Moreover, handle errors gracefully; write code that anticipates potential problems, and offer clear solutions when things go wrong.
Regularly refactor your code to improve its structure and readability, and use version control systems like Git to track changes and facilitate collaboration among developers.
Also Explore: Top 10 Tools Every Full-Stack Developer Should Master in 2024
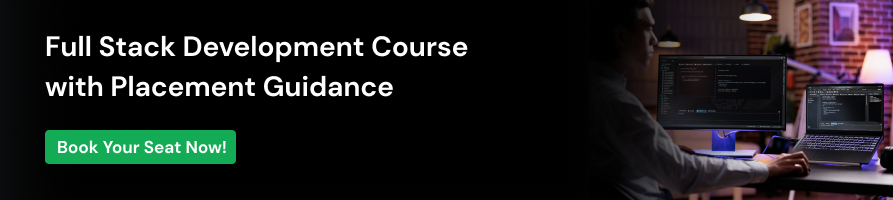
Best Practices for Writing Clean Code
Now that we’ve understood what the key principles are, let us learn about some practices that will help you maintain those principles:
1) Meaningful Naming and Function Management
To foster readability and maintainability in your code, start with using descriptive and unambiguous names for variables, functions, and classes.
Opt for names that reflect their purpose—like calculateTotal
instead of func1
.
This clarity extends to keeping functions compact and focused on a single task, which simplifies understanding and testing. For instance, avoid creating multi-purpose functions that handle more than one operation.
Also Read: “var functionName = function” VS “function functionName”
2) Strategic Use of Comments and Documentation
While clean code should largely speak for itself, judicious use of comments can clarify the intent behind complex logic or important decisions.
Aim to write comments that explain “why” something is done, not “how” it’s done—the code itself should be clear enough for the latter.
Ensure all documentation is up-to-date and directly relevant, removing any outdated or redundant comments that could mislead other developers.
3) Consistency and Code Structure
Adhere to a consistent coding style across your entire project. This includes consistent formatting, indentation, and organizing code blocks logically.
Use tools like linters or formatters to automate and enforce these standards. Emphasize the DRY (Don’t Repeat Yourself) principle to avoid redundancy, which can reduce maintenance overhead and improve code clarity.
Regular refactoring and code reviews should be integral to your development process, helping to maintain a clean and efficient codebase that is easier for you and your team to work with over time.
Also Know About 5 Best Websites to Practice Coding for Competitive Programming
Common Pitfalls to Avoid
Avoiding common pitfalls in full stack development is crucial for maintaining the integrity and efficiency of your code. Here are some critical areas to watch for:
1) Insufficient Planning and Design
Failing to define clear objectives and architecture can lead to a convoluted codebase that is hard to maintain and scale. Ensure you spend adequate time in the planning phase to set a solid foundation for your project.
2) Ignoring Code Readability and Maintainability
Code that is difficult to read or understand becomes a significant barrier to future development. Strive for simplicity and clarity in your coding practices, and always consider the next developer who might work on your code.
3) Not Handling Errors and Exceptions Properly
Proper error and exception handling are essential for creating reliable and robust applications. Ensure your code gracefully handles unexpected situations to prevent crashes and undesirable behavior.
4) Overlooking Security Vulnerabilities
Security is a paramount concern in development. Neglecting to implement security measures can expose your application to attacks and data breaches. Regularly update your security practices and audit your code for vulnerabilities.
5) Poor Version Control Practices
Version control is vital for effective team collaboration and maintaining the history of your code changes. Poor practices can lead to code conflicts and loss of work. Utilize tools like Git to manage your codebase efficiently.
Know More About Top 10 Full-Stack Developer Frameworks in 2024
By steering clear of these pitfalls, you can enhance the quality and reliability of your software, ensuring it not only meets the current requirements but is also robust and maintainable for future enhancements.
Tools and Techniques for Maintaining Clean Code
Identify and Implement Effective Tools and Techniques
- Design Patterns and Frameworks:
- Before coding begins, select appropriate design patterns and frameworks that align with project requirements and team skills.
- Establish agreed-upon standards and conventions to ensure consistency throughout the development process.
- Utilize flexible architectural runways to accommodate future changes without significant rework.
- Version Control Systems:
- Implement robust version control systems like Git to manage changes and collaborate effectively.
- Regularly commit changes to document the development process and facilitate rollback if necessary.
- Refactoring and Testing Tools:
- Integrate refactoring tools available in IDEs such as IntelliJ and Visual Studio to streamline code improvements.
- Leverage testing tools to automate testing processes, ensuring code functionality and reducing bugs.
Emphasize Code Quality and Maintenance
- Regular Code Refactoring:
Regularly revisit and refine code to adapt to new requirements or feedback. This practice not only improves code quality but also extends its life. - Effective Error Handling:
Develop a standard error code and message list for the application to standardize responses and avoid exposing sensitive information. - Documentation and Comments:
Maintain up-to-date documentation and use comments judiciously to explain the ‘why’ behind complex logic, enhancing understandability and maintainability.
Also Read: Best Ways to Learn Full Stack Development in 2024
Utilize Advanced Development Practices
- Test-Driven Development (TDD):
Adopt TDD to ensure that all new code is covered by tests, which helps to catch issues early and design better software architectures. - Self Code Reviews:
Before integrating changes, conduct self-reviews to catch potential issues and ensure adherence to coding standards. - Continuous Integration and Testing:
Set up continuous integration tools to automate building and testing code changes, which helps in identifying integration issues early.
Leveraging Version Control for Collaboration
Version Control Systems (VCS) are indispensable in managing changes and fostering collaboration within software development teams. By using tools like Git, developers can effectively track and manage modifications to the codebase, ensuring that all team members are aligned and informed. Here’s how version control enhances collaboration:
Key Concepts of Version Control
- Commits and Repositories: Commits act as snapshots of your project at specific points, allowing you to track changes and revert to previous versions if needed. Repositories serve as central hubs where all project files and their histories are stored.
- Branches and Merging: Developers use branches to work on new features or fixes without affecting the main codebase. Merging integrates these changes back, after a thorough review through pull requests, ensuring that modifications are sound before they become part of the main project.
- History and Timeline: This feature records every change made to the codebase, who made it, and when it was made, which is crucial for understanding the evolution of a project and resolving disputes or confusion about changes.
Collaborative Tools and Practices
- Centralized Repository: A shared repository on platforms like GitHub or GitLab allows team members to push their changes, submit pull requests, and review code collectively.
- Continuous Integration/Continuous Deployment (CI/CD): These practices involve automated testing and deployment of code changes, helping teams to detect issues early and streamline the release process.
- Communication Tools: Integration with tools like Slack or Jira facilitates communication regarding code changes, feature requests, and bug reports, keeping everyone on the same page.
Best Practices for Collaborative Development Using Git
- Frequent Commits: Regular commits with clear, descriptive messages provide a detailed history of changes and simplify troubleshooting and understanding of the code evolution.
- Branching Strategy: Employ a consistent branching strategy, like Git Flow, to manage features, fixes, and releases, which helps in organizing work and reducing conflicts.
- Code Reviews: Before merging, conduct code reviews to ensure quality, share knowledge among team members, and maintain a high standard of code health.
By leveraging these version control practices, teams not only improve their operational efficiency but also enhance the quality and reliability of their software products.
Also Read: Full Stack Developer Roadmap: A Complete Guide [2024]
Error Handling and Code Robustness
1) Implementing Effective Error Handling Strategies
To ensure your applications are robust and user-friendly, it’s crucial to implement comprehensive error-handling strategies.
Start by using try-catch blocks to manage exceptions gracefully. This prevents errors from crashing your application and allows for a controlled response to unexpected issues.
For each catch block, provide informative error messages that guide users or developers on how to resolve the issue or where to find more help.
2) Comprehensive Logging and Exception Management
Effective logging is essential for diagnosing problems quickly. Log not only the error messages but also stack traces and contextual data that can help in troubleshooting.
Adopt a standardized approach to logging across your codebase to maintain readability and ease collaboration. Additionally, create a hierarchy of application-specific exceptions to better categorize errors and handle them more effectively.
This structured approach aids in maintaining clean code and enhances maintainability.
3) Continuous Improvement of Error Handling Practices
Regularly review and refine your error-handling strategies to adapt to new challenges and feedback from testing and production environments.
Implement automated testing to cover various error scenarios, ensuring your application responds correctly under different conditions.
Use feedback from these tests to iterate on your error-handling methods, making your software more resilient against future issues.
Continuously improving these practices is key to developing stable and reliable software systems.
Also Read: 5 Career Opportunities for Full Stack Development
Implementing Refactoring and Testing
Refactoring is a systematic process aimed at improving code quality without adding new functionality. It involves a meticulous approach where each change is followed by testing to ensure functionality remains intact. Here’s a step-by-step guide to effective refactoring:
- Assess and Plan: Begin by identifying sections of the code that are complex, hard to understand, or not performing optimally. Plan your refactoring steps carefully, ensuring each change is small and manageable.
- Execute Refactoring: Implement refactoring techniques such as ‘Extract Method’ or ‘Branch by Abstraction’. These methods help simplify and clarify the code, making it easier to maintain and extend.
- Test Rigorously: After each refactoring step, run your suite of automated tests to confirm that the functionality is preserved. This is crucial as it prevents new bugs from being introduced during the refactoring process.
Refactoring also includes continuous monitoring and improvement of the codebase. It’s essential to document the changes made during refactoring for future reference and to aid other developers working on the project. Regularly updating the documentation ensures that the rationale behind code changes is clear, promoting better understanding and easier maintenance.
Also Find Out Software Testing vs. Quality Assurance (QA)
Moreover, refactoring should be supported by robust testing practices. Implementing Test-Driven Development (TDD) can be particularly effective.
In TDD, tests are written before the code itself, which ensures that the software is designed to meet its requirements from the outset. This not only enhances code quality but also reduces the likelihood of bugs and improves maintainability over time.
Finally, it’s important to engage in refactoring as a regular practice, integrated into the development cycle. By making refactoring a routine part of development, you can maintain a clean, efficient codebase that is easier to adapt as new requirements arise or as the technology landscape evolves.
Concluding Thoughts…
Throughout this guide, we’ve navigated the intricacies of applying clean code principles within full stack development, underscoring their paramount importance for enhancing maintainability, readability, and overall collaborative efficacy in the development process.
Clean code principles, from employing meaningful names to embracing the DRY principle and leveraging version control for effective collaboration, have been illustrated as foundational to building maintainable, readable, and functional applications.
The emphasis on simplicity and consistency across coding practices further fortifies the software development lifecycle, ensuring that applications not only meet current demands but are also poised for future adaptations with minimal friction.
Also Read: Exploring the Influence of AI and Machine Learning in Full Stack Development [2024]
FAQs
What are the fundamental principles of clean code?
Clean code adheres to four main principles: it must successfully pass all tests, clearly reveal its intentions, minimize duplication, and use the fewest elements necessary to achieve its purpose.
Is the “Clean Code” book suitable for beginner programmers?
Yes, “Clean Code” is highly recommended for beginners. It provides a solid foundation for writing clean code before moving on to more advanced topics like those covered in “Code Complete,” which deals with broader software development processes and might be overwhelming for someone new to programming.
Did you enjoy this article?