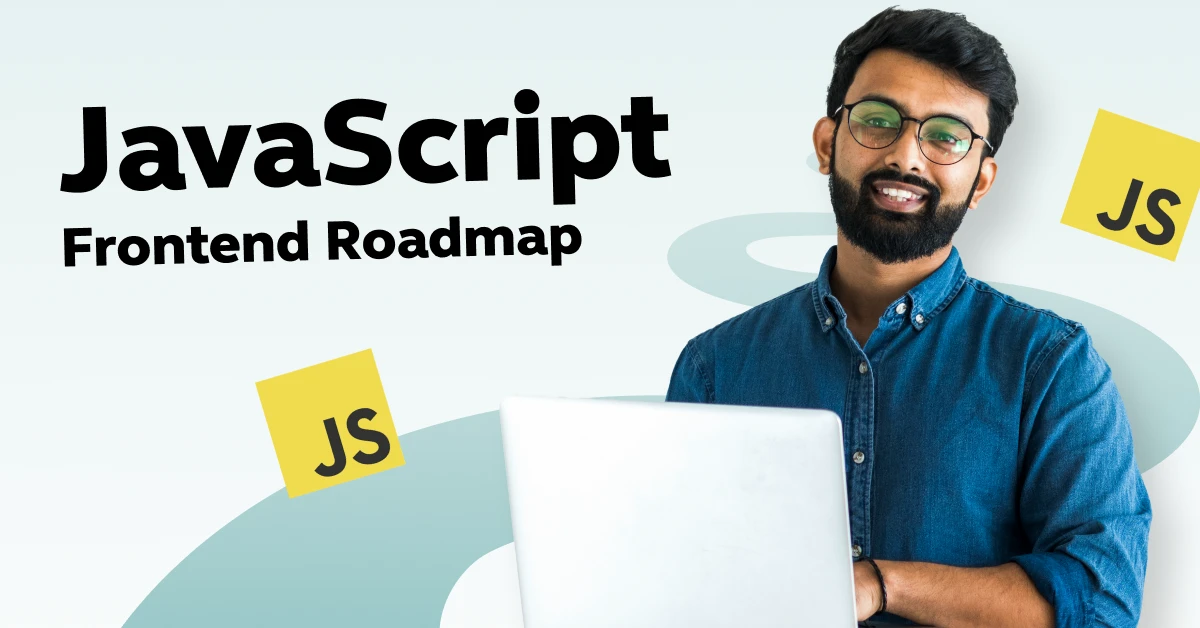
Master JavaScript Frontend Roadmap: From Novice to Expert [2024]
Mar 22, 2024 7 Min Read 2083 Views
(Last Updated)
Are you eager to learn JavaScript? Want to dive deep into the JavaScript frontend roadmap? Want to know what are the skills and technologies required to become a front-end developer?
So many doubts, right? Calm down! All of your doubts will be answered here. We all get fascinated by those buttons, forms, menus, and pages on our screen when we look for any general query on the internet. Those are created by none other than front-end developers.
Front-end development is the creation of a webpage that has an engaging and intuitive user experience. When that front end is built using JavaScript, it helps us create very dynamic and interactive user interfaces. We have created this blog for front-end developers who want to build interactive websites using JavaScript. Also, no matter whether you’re a novice or an experienced developer, this blog covers every minute detail of things you should know.
Welcome to this blog post on the frontend roadmap in JavaScript!
Let us go step-by-step:
Table of contents
- HTML and CSS
- a. Getting Started
- b. HTML Tags and Attributes
- c. CSS Selectors and Properties
- d. Layout and Positioning
- Fundamentals of JavaScript
- a. Variables and Data Types
- b. Operators and Expressions
- c. Control Structures
- d. Functions and Scope
- e. Arrays and Objects
- Modern JavaScript Concepts
- a. ES6 Syntax and Features
- b. Asynchronous Programming with Promises and Async/Await
- c. DOM Manipulation with jQuery
- d. Modular Code Organization with Modules and Bundlers
- Frontend Frameworks
- JavaScript Libraries and Frameworks
- a) Libraries and Frameworks
- b) Choosing the Right Library or Framework for a Project
- Building and Deploying Applications
- a. Setting Up a Development Environment
- b. Using Build Tools like Webpack and Gulp
- c. Deploying Applications to Production
- Best Practices and Emerging Trends
- Conclusion
- FAQs
- Q1. What is JavaScript Frontend development?
- Q2. How long does it take to learn front-end development?
- Q3. What skills are required for JavaScript Frontend development?
- Q4. How much JavaScript is enough for front-end development?
1. HTML and CSS
HTML and CSS are essential technologies for creating beautiful and responsive websites. This section will explore the basics of HTML and CSS, including the key concepts and features that make up these technologies.
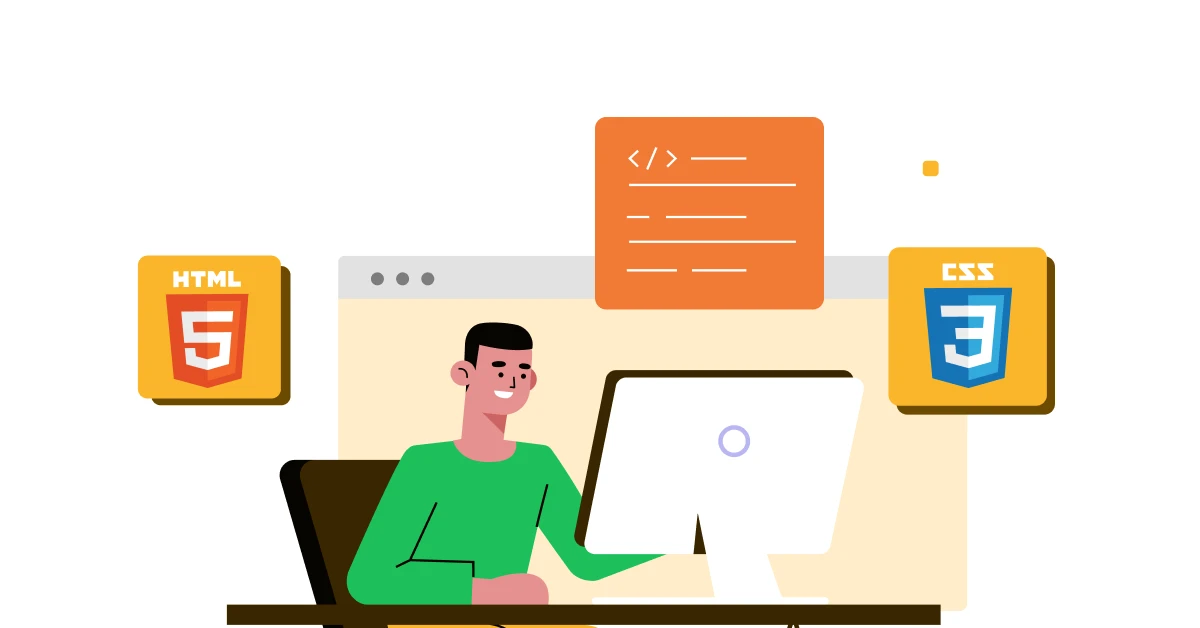
a. Getting Started
HTML is the standard markup language used to create the structure and the content of web pages. CSS (Cascading Style Sheets) is a stylesheet language used to control the visual presentation of HTML documents. HTML and CSS provide the foundation for creating visually appealing and responsive websites.
HTML and CSS collectively bring life to the webpage with elements and colors!
b. HTML Tags and Attributes
HTML documents comprise a series of tags, which define the structure and content of the page. The tags are enclosed in angle brackets and can include attributes that provide additional information about the tag. Some of the most common HTML tags include <html>, <head>, <title>, <body>, <div>, <p>, <img>, <a>, and <form>.
c. CSS Selectors and Properties
CSS uses selectors to target specific HTML elements and apply styles to them. Selectors can be based on element types, classes, IDs, and other attributes. CSS properties control the appearance of HTML elements, such as color, font, size, spacing, and layout. The most common CSS properties include color, font family, font size, margin, padding, and display.
d. Layout and Positioning
Layout and positioning are crucial aspects of CSS that help create visually appealing and responsive web pages. CSS provides several methods for positioning HTML elements, including static, relative, absolute, and fixed. CSS also provides tools for creating responsive layouts, such as Flexbox and Grid.
Before diving into the next section, ensure you’re solid on full-stack development essentials like front-end frameworks, back-end technologies, and database management. If you are looking for a detailed Full Stack Development career program, you can join GUVI’s Full Stack Development Career Program with placement assistance. You will be able to master the MERN stack (MongoDB, Express.js, React, Node.js) and build real-life projects.
Instead, if you would like to explore HTML and CSS through a Self-paced course, try GUVI’s Modern HTML and CSS Self-Paced certification course.
2. Fundamentals of JavaScript
JavaScript is a powerful and versatile programming language widely used in frontend and backend web development. To become proficient in JavaScript, it is essential to understand the fundamental concepts and features of the language. This section will explore the key topics that comprise the fundamentals of JavaScript.
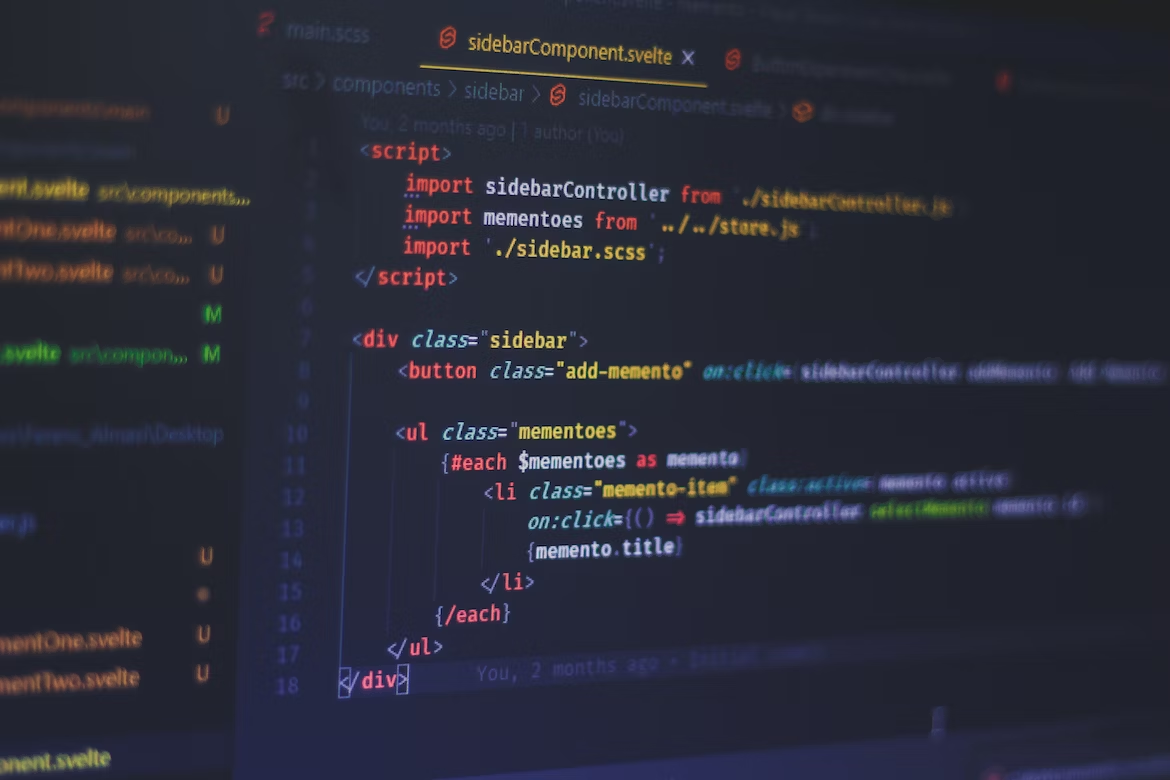
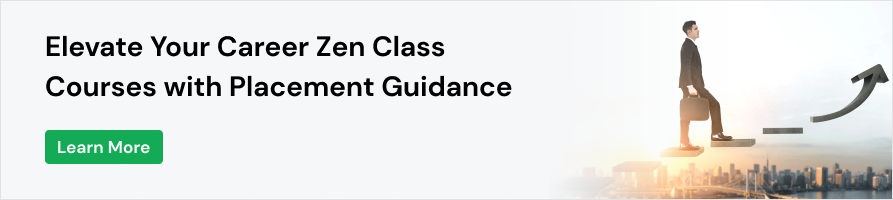
a. Variables and Data Types
Variables are used in JavaScript to store and manipulate data. A variable is declared using the “let” or “const” keyword, followed by a name and an optional initial value. JavaScript supports several data types: strings, numbers, booleans, null, and undefined. Variables can also hold complex data types, such as arrays and objects.
b. Operators and Expressions
Operators are used in JavaScript to perform arithmetic, comparison, and logical operations on values. JavaScript supports a range of operators, including arithmetic operators (+, -, *, /), comparison operators (>, <, >=, <=), and logical operators (&&, ||, !). Expressions are combinations of variables, operators, and values that evaluate a single value.
c. Control Structures
Control structures are used in JavaScript to control the flow of code execution based on conditions. JavaScript supports several control structures, including “if” statements, “switch” statements, “for” loops, and “while” loops.
d. Functions and Scope
Functions are reusable code blocks that perform a specific task. In JavaScript, functions are declared using the “function” keyword, followed by a name and a set of parameters. Functions can also return values and can be used to manipulate variables and data.
The scope is the visibility of the variables within a program. In JavaScript, variables declared inside a function are only visible within that function, while variables declared outside a function are visible throughout the program.
e. Arrays and Objects
Arrays and objects are complex data types that allow developers to store and manipulate multiple values in a single variable. Arrays are used to store the collection of values, while objects are used to store key-value pairs. JavaScript provides a range of methods and properties to manipulate arrays and objects, making them powerful and versatile data types.
By mastering the fundamentals of JavaScript, you can lay a solid foundation for your journey as a front-end developer. These concepts are essential to understanding more advanced JavaScript topics and frameworks like React and Angular. So, take the time to practice and experiment with these concepts to become a confident and skilled JavaScript developer.
3. Modern JavaScript Concepts
Modern JavaScript concepts have revolutionized the way developers write front-end code. This section will explore some essential concepts, including ES6 syntax and features, asynchronous programming with Promises and Async/Await, DOM manipulation with jQuery, and modular code organization with modules and modules and bundlers.
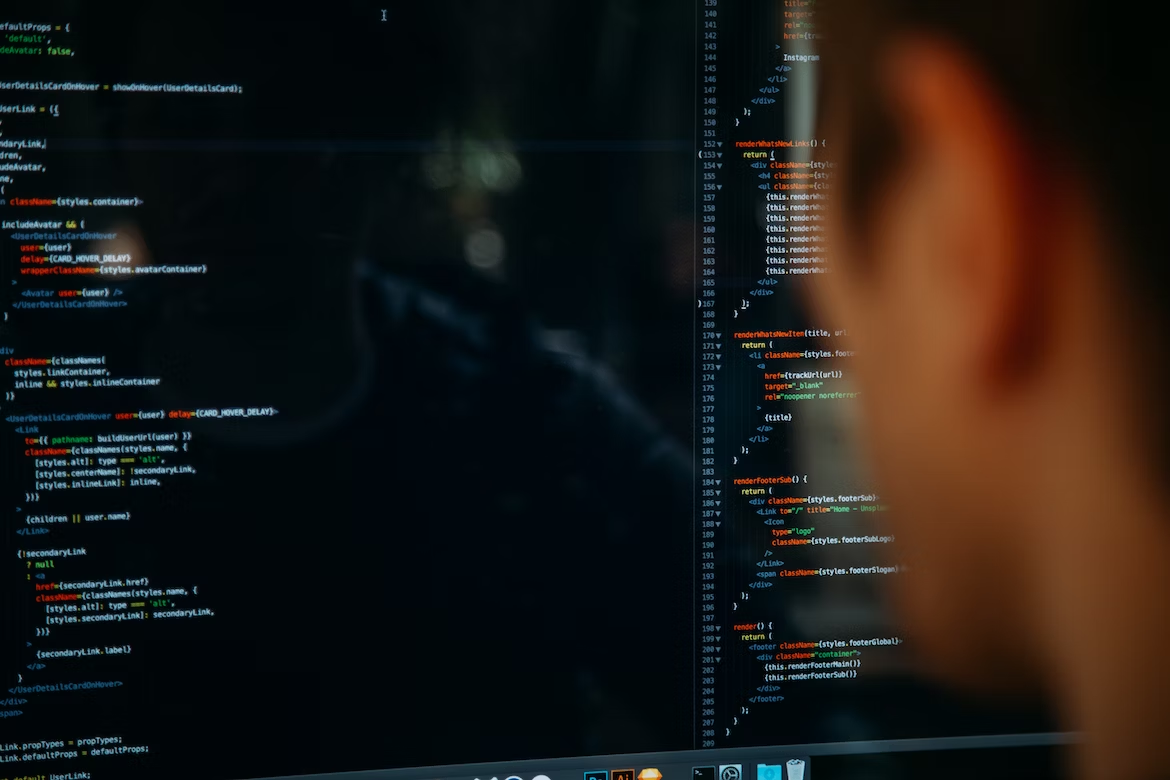
a. ES6 Syntax and Features
ES6 (ECMAScript 6) introduced several new syntax features that make JavaScript code more concise and easier to read. The most important features include arrow functions, template literals, destructuring, rest and spread operators, and classes. By mastering these features, developers can write cleaner, more efficient code that is easier to maintain and understand.
b. Asynchronous Programming with Promises and Async/Await
Asynchronous programming is crucial for building responsive web applications that handle complex user interactions and data requests. Promises and Async/Await are two modern techniques for managing asynchronous code in JavaScript. Promises provide a way to handle asynchronous operations more elegantly and predictably, while Async/Await simplifies syntax and makes it easier to read and write asynchronous code.
c. DOM Manipulation with jQuery
jQuery is a trendy JavaScript library that provides a concise and easy-to-use API for manipulating the Document Object Model (DOM). With jQuery, developers can easily add and remove elements, modify CSS styles, handle events, and perform animations, among other things. While jQuery has become less necessary in recent years with the rise of modern frameworks like React and Vue, it is still an essential tool for many front-end developers.
d. Modular Code Organization with Modules and Bundlers
Modular code organization is critical for building large-scale web applications that are easy to maintain and update. ES6 introduced a standardized module syntax that allows developers to break their code into separate modules that can be imported and exported as needed.
Bundlers like Webpack and Rollup provide tools for combining these modules into a single file the browser can load. By using modules and bundlers, developers can create modular and efficient code that is easier to test, maintain, and deploy.
Modern JavaScript concepts like ES6 syntax and features, asynchronous programming with Promises and Async/Await, DOM manipulation with jQuery, and modular code organization with modules and bundlers have revolutionized the way developers write frontend code. By mastering these concepts, developers can create robust and efficient web applications that meet the requirements and needs of their users.
We have curated these 2 JavaScript courses which are very pocket-friendly and will act as a bonus point to your JavaScript Frontend Roadmap:
1. JavaScript course which will not only help you learn concepts but will also be awarded a globally recognized, job-ready certificate.
2. Advanced JavaScript course which will help you learn more advanced features and you’ll be able to create more complex and responsive JavaScript code, making you a sought-after JavaScript developer.
4. Frontend Frameworks
Frontend frameworks are essential tools for building complex and dynamic web applications. Frontend frameworks are pre-written code libraries that developers can use to build web applications more quickly and easily. They provide a set of pre-built components and features that can be easily customized and integrated into a project.
In this section, we will explore what frontend frameworks are, provide an overview of some of the most popular frameworks, including React, Angular, and Vue.js, and help you understand their differences.
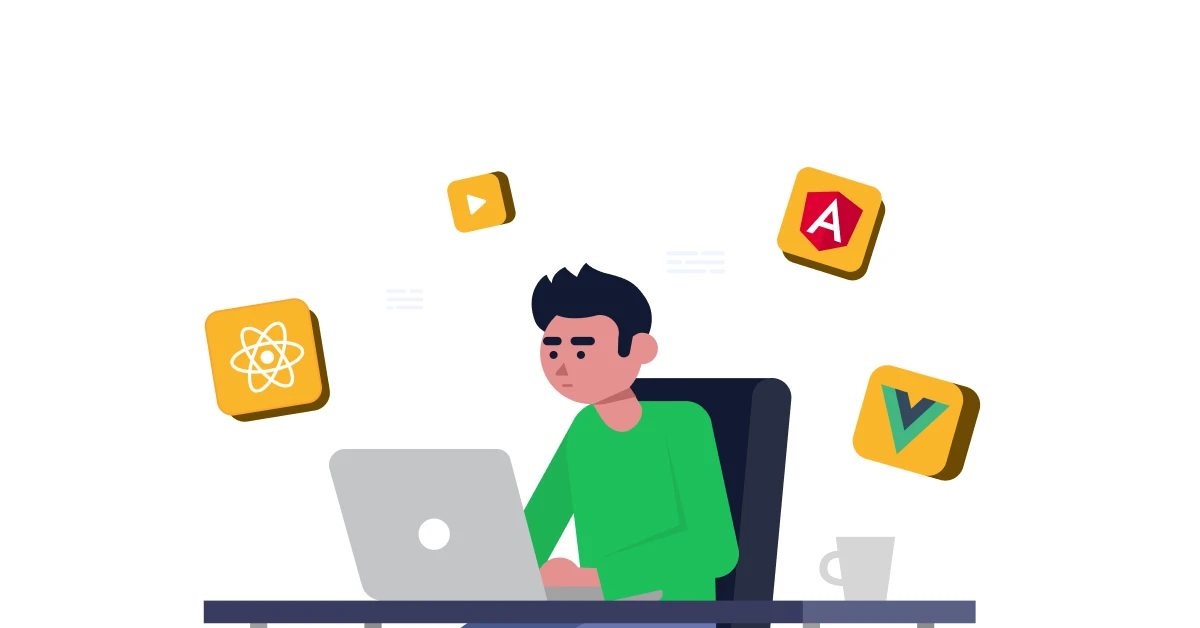
1. React – React is a popular open-source JavaScript library for building user interfaces. Facebook maintains it and has a large and active community of developers. React uses a virtual DOM to update the user interface efficiently, which results in faster and smoother performance. It is highly modular and flexible, making it an excellent choice for building large and complex applications.
2. Angular – Angular is a front-end framework developed and maintained by Google. It provides a complete toolkit for building complex web applications, including powerful data binding, component architecture, and testing tools. Angular is highly opinionated and requires developers to follow specific coding patterns, which can make it more challenging to learn but also ensures consistency and maintainability.
3. Vue.js – Vue.js is a progressive JavaScript framework designed to be easy to learn and use. It is highly modular and allows developers to start small and gradually add more complex functionality as needed. Vue.js is well known for its simplicity and flexibility and has a growing community of developers.
Also Read: React vs Angular vs Vue: Choosing the Right Framework [2024]
5. JavaScript Libraries and Frameworks
JavaScript libraries and frameworks are essential for front-end developers, allowing them to quickly and efficiently build complex web applications. This section will explore the basics of JavaScript libraries and frameworks and some of the most popular options available.
a) Libraries and Frameworks
JavaScript libraries and frameworks are pre-written code libraries that provide a set of functionalities and features that developers can use to create web applications. Libraries typically focus on tasks such as DOM manipulation, animation, or AJAX requests, while frameworks provide a more comprehensive structure for building web applications.
Popular JavaScript Libraries and Frameworks
Many popular JavaScript libraries and frameworks are available, each with strengths and weaknesses. Some of the most widely used libraries include
- jQuery
- Lodash
- D3.js
b) Choosing the Right Library or Framework for a Project
Several factors must be considered when selecting a JavaScript library or framework for a project. These include the project’s requirements, the level of community support and documentation, the learning curve for the library or framework, and the compatibility with other tools and technologies used in the project.
By carefully considering these factors, developers can choose the right tool for the job and create high-quality web applications efficiently and effectively.
6. Building and Deploying Applications
Building and deploying applications is an essential aspect of front-end development. This section will cover setting up a development environment, using build tools like Webpack and Gulp, and deploying applications to production.
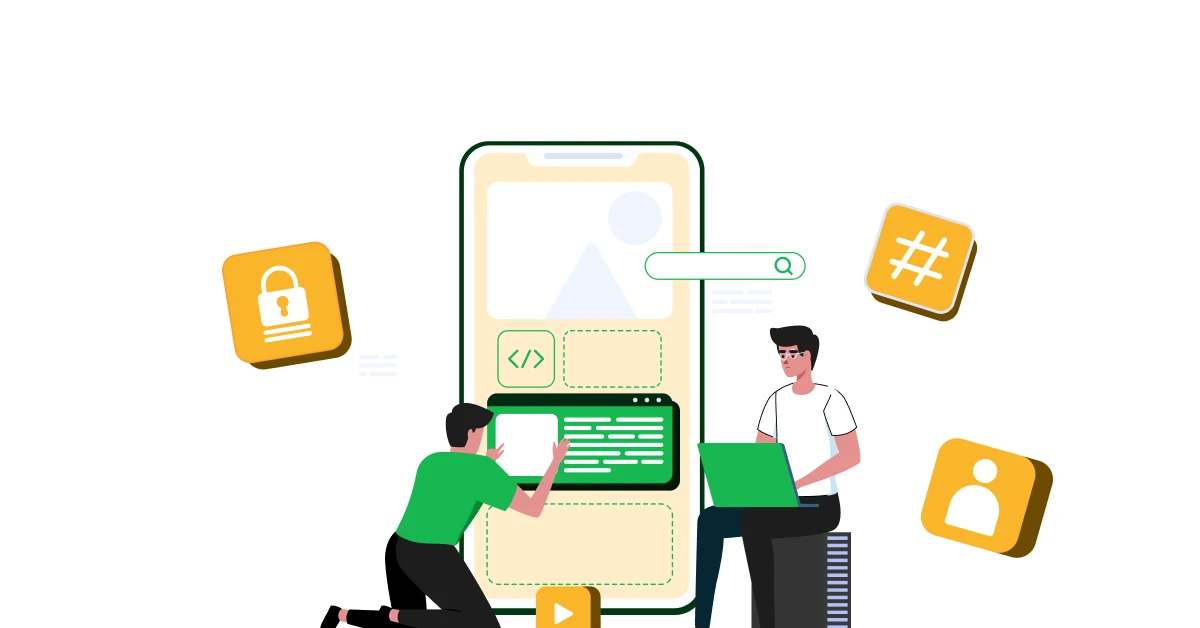
a. Setting Up a Development Environment
Before building a web application, you must set up your development environment. This includes installing the necessary software and tools, such as a code editor, web browser, and version control system. You may also need to install additional dependencies, such as a package manager like npm or yarn.
b. Using Build Tools like Webpack and Gulp
Build tools like Webpack and Gulp are used to automate the build process and make it easier to manage dependencies, optimize code, and generate production-ready assets. Webpack is a popular build tool that can handle various tasks, including bundling modules, optimizing images and CSS, and running tests. Gulp is another popular build tool that allows you to automate tasks using simple and flexible code.
c. Deploying Applications to Production
Deploying a web application to production can be a complex process, but it is very essential to ensure that your application is accessible and reliable for users. Depending on the hosting environment, you may need to set up a server, configure SSL certificates, and deploy your application using a tool like FTP or SSH. You may also need to set up a CI/CD pipeline to automate the deployment process and ensure that new updates are automatically deployed to production.
Building and deploying web applications is critical to front-end development. By setting up a development environment, using build tools like Webpack and Gulp, and deploying applications to production, you can ensure your application is optimized, reliable, and accessible to users.
Do try working on some of the best JavaScript project ideas to get your hands-on practice.
7. Best Practices and Emerging Trends
As front-end development in JavaScript evolves, staying up-to-date with the latest best practices and emerging trends is essential. This section will cover some best practices and emerging trends in front-end development.
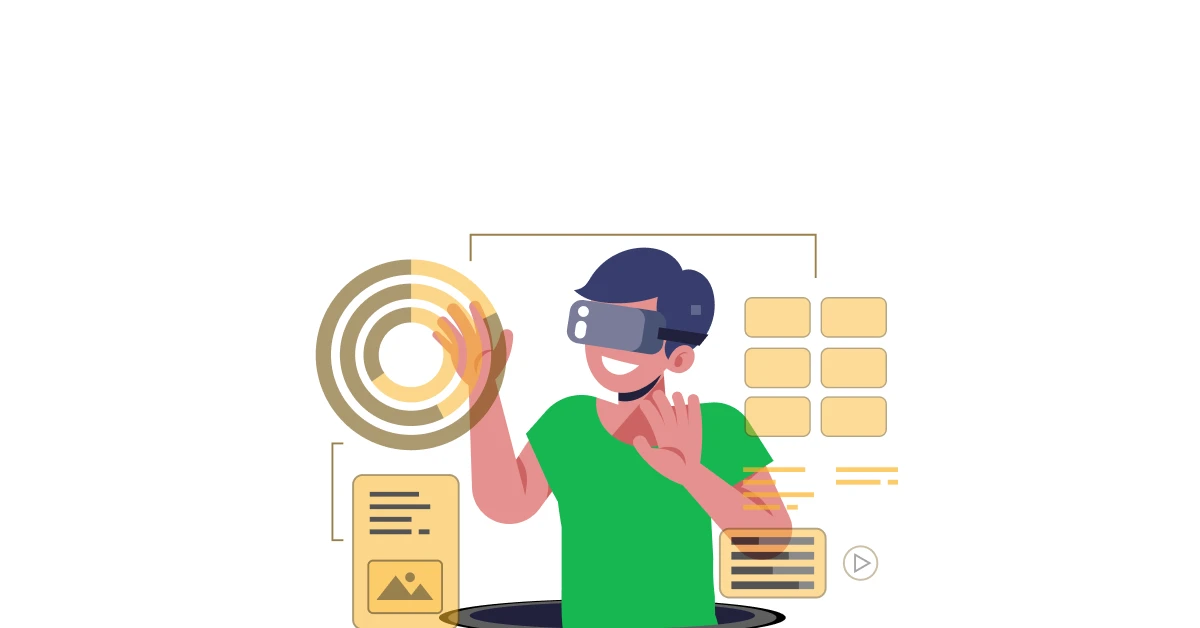
Best Practices for Frontend Development in JavaScript:
- Write clean and maintainable code by following best practices and principles like SOLID and DRY.
- Use version control to manage code changes and collaborate with team members.
- Optimize performance by minimizing external dependencies, optimizing images and code, and using lazy loading techniques.
- Ensure accessibility by following best practices for web accessibility, such as using semantic HTML and ARIA attributes.
- Test code thoroughly using automated testing frameworks and manual testing.
- You should have a habit of building projects on frontend development which will help you get a deeper understanding.
Latest Trends in Frontend Development
a) Serverless Architectures
Serverless architectures are becoming increasingly popular in front-end development. They allow you to focus on writing code without worrying about server infrastructure, scaling, and management.
b) Progressive Web Apps
Progressive Web Apps (PWAs) provide a mobile-app-like experience for users while still being accessible through a web browser. PWAs can be installed on the user’s device and offer features such as offline access and push notifications.
c) Static Site Generators
Static site generators like Gatsby and Next.js are becoming popular for building fast, scalable, and secure web applications. They allow you to build a static website and then dynamically generate pages using APIs and data sources.
Following best practices and staying up-to-date with emerging trends in front-end development can help you build better web applications. By writing clean and maintainable code, optimizing performance, ensuring accessibility, and keeping up with the latest trends, you can create fast, scalable, and user-friendly applications.
Kickstart your Full Stack Development journey by enrolling in GUVI’s certified Full Stack Development Career Program with placement assistance where you will master the MERN stack (MongoDB, Express.js, React, Node.js) and build interesting real-life projects. This program is crafted by our team of experts to help you upskill and assist you in placements.
Instead, if you would like to explore HTML and CSS through a Self-paced course, try GUVI’s Modern HTML and CSS Self-Paced certification course.
Conclusion
Now that you have gone through this JavaScript Frontend Roadmap, it’s also important to stay updated with the current trends and technologies. You can also join online communities like Stack Overflow, Reddit, or GitHub to connect with other developers and ask questions.
Experiment with the new technologies and push the boundaries of what is possible in the JavaScript frontend roadmap. By following the JavaScript frontend roadmap and putting it into practice, you can build web applications that are optimized, scalable, and user-friendly.
FAQs
Q1. What is JavaScript Frontend development?
Ans. JavaScript front-end development refers to the part of a web application that runs in the user’s browser and is responsible for the user interface and user experience. JavaScript is a programming language that is commonly used for front-end development and is used to create dynamic, interactive web pages.
Q2. How long does it take to learn front-end development?
Ans. It takes three to six months for students to learn front-end development with a set of industry-level skills. It would be best if you enriched yourself with frameworks, and libraries, and worked on building portfolios.
Q3. What skills are required for JavaScript Frontend development?
Ans. JavaScript Frontend development requires:
1. Strong understanding of HTML, CSS, and JavaScript
2. Knowledge of frontend frameworks and libraries
3. Responsive design principles
4. Web performance optimization.
5. VCS
Did you enjoy this article?