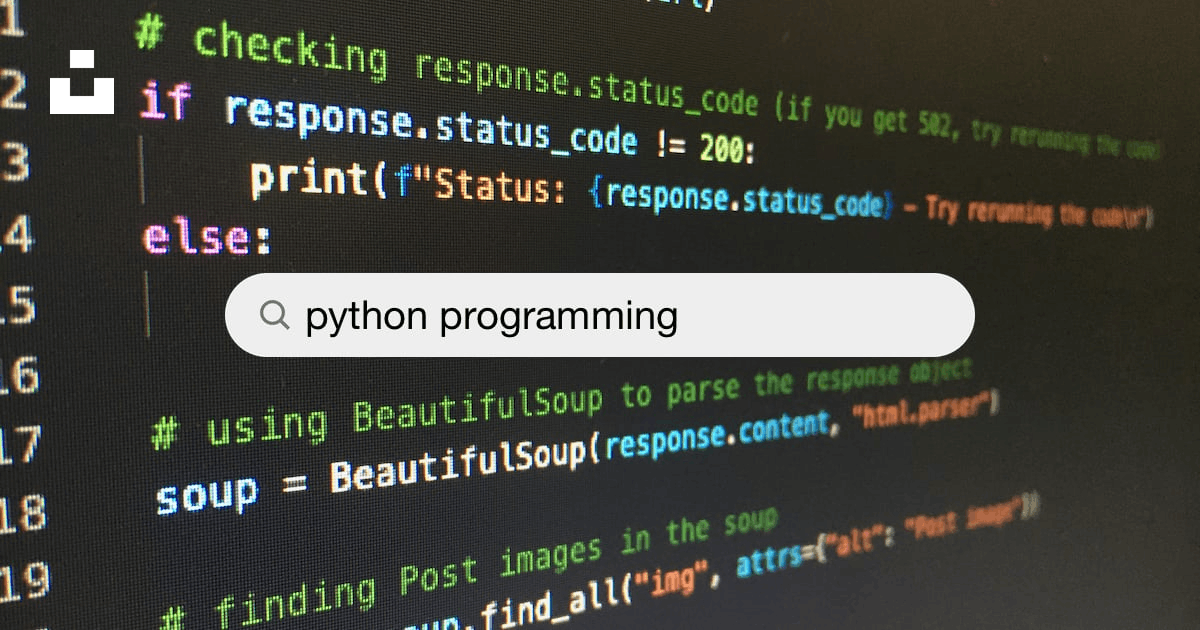
Python Objects 101: How to Create and Master Them With Real-World Projects
Apr 16, 2024 6 Min Read 435 Views
(Last Updated)
As a keen Python developer, I am a die-hard fan of ‘objects’ as they have made building complex functions so much fun for me. Really, they make coding life so easy and simple, like they’re one of the most prominent reasons why Python is such a beginner-friendly language.
And why you must know them? Well, the rising popularity of Python in the tech world is nothing new as the language basically has its hands dipped in all of the major development sectors, be it Web, Data Science, Machine Learning, Artificial Intelligence, the list goes on and on. Just to give you a gist, read this news article by TOI as to what makes Python a necessary and critical skill for IT Professionals.
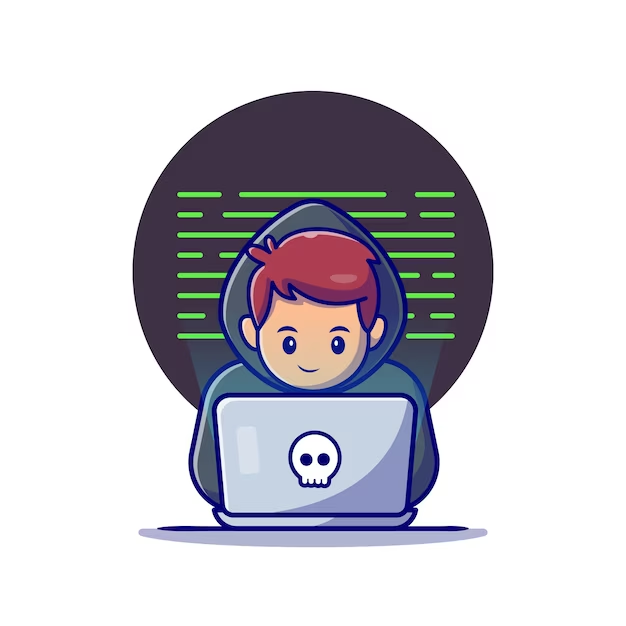
So, we thought we’d give you a headstart on one of the most intriguing and must-know concepts of this in-demand language so that you can begin your learning journey with ease.
Python objects are incredibly powerful and flexible, allowing developers to create complex applications with ease. In this article, I will be discussing the basics of Python objects, how to create and implement them in real-life projects, and the best practices for doing so.
Table of contents
- Introducing Python Objects
- Understanding Classes and Objects in Python
- Defining Attributes and Methods in Classes
- Creating Objects in Python
- Initializing and Modifying Python Objects
- Must-Know Python Class Concepts
- 1)Inheritance in Python Classes
- 2)Polymorphism in Python Classes
- Encapsulation in Python Objects
- 1)Private attributes and methods
- 2)Protected attributes and methods
- Top Use Cases for Python Objects
- Best Practices for Creating and Implementing Python Objects
- Implementing Python Objects in Real-Life Projects
- Concluding Thoughts...
Introducing Python Objects
At its very core, Python is an object-oriented programming language. This means that everything in Python is an object. An object is an instance of a class, which is a blueprint that defines the properties and methods of an object (basically what the object will represent and do).
Objects in Python are used to represent and manipulate data. They are created using classes as we discussed above, which define their properties and methods.
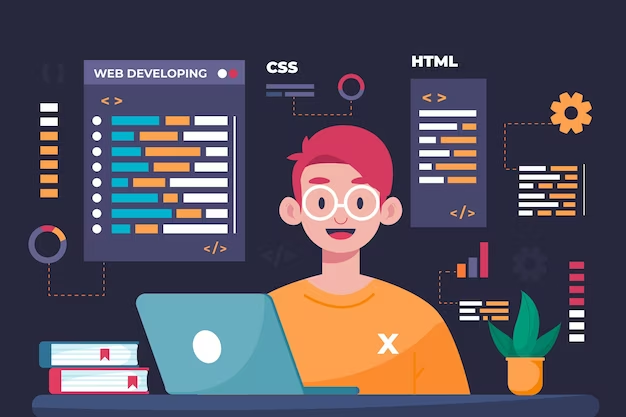
In Python, a class is defined using the ‘class’ keyword, followed by the name of the class. For example, a simple class definition in Python would look like this:
class MyClass:
pass
This creates a new class called MyClass that does nothing. However, we can now create instances of this class, or objects, using the following code:
my_object = MyClass()
This creates a new instance of the MyClass class and assigns it to the variable my_object.
By using objects, we can organize and structure our code in a more efficient and modular way, making it easier to read and maintain. Objects also allow us to reuse code and create complex systems by combining different classes.
Overall, objects are an essential feature of Python and many other programming languages.
Before diving into the next section, ensure you’re solid on Python essentials from basics to advanced level. If you are looking for a detailed Python career program, you can join GUVI’s Python Career Program with placement assistance. You will be able to master the Multiple Exceptions, classes, OOPS concepts, dictionary, and many more, and build real-life projects.
Also, if you would like to explore Python through a Self-paced course, try GUVI’s Python Self-Paced course.
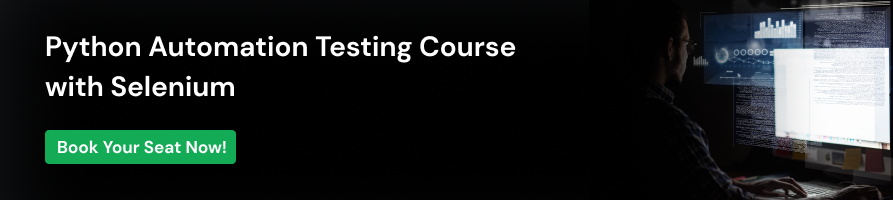
Understanding Classes and Objects in Python
As mentioned earlier, a class is like a blueprint that defines what the object does. In Python, a class can have attributes and methods. Attributes are variables that hold data and methods are functions that perform actions on the data.
For example, let’s create a new class called Person that has two attributes, name and age, and a method called speak that prints out a greeting:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def speak(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
Here, we have defined a constructor method called __init__, which is called when a new instance of the class is created. This method takes two arguments, name, and age, and assigns them to the name and age attributes of the object.
We have also defined a method called speak, which prints out a greeting using the name and age attributes that we earlier defined. See? That’s how easy it is with objects, you create them and then use them wherever you like (following the scope of the class ofcourse).
Defining Attributes and Methods in Classes
I just gave you a gist about these aspects in the sub-section above. Now, let’s go a little deeper.
Attributes and methods are the building blocks of Python classes. Attributes are variables that hold data and methods are defined functions that perform certain action on the data.
To define attributes in a class, we simply create variables inside the class definition. For example, here’s how we can define the name and age attributes in the Person class:
class Person:
name = ""
age = 0
To define methods in a class, we create functions inside the class definition. For example, here’s how we can define the speak method in the Person class:
class Person:
name = ""
age = 0
def speak(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
Methods can take arguments just like regular functions. For example, here’s how we can modify the speak method to take a greeting argument:
class Person:
name = ""
age = 0
def speak(self, greeting):
print(f"{greeting}, my name is {self.name} and I am {self.age} years old.")
Just try these yourself, and you’ll see the differences with each set of code.
Creating Objects in Python
Its pretty simple, in fact we’ve already covered it above, just not explicitly.
To create an object in Python, we use the class keyword followed by the name of the class since they can only be defined in classes. We can then set the attributes of the object using the ‘dot’ notation. For example, let’s create a new Person object called john:
class Person:
name = ""
age = 0
john = Person()
john.name = "John"
john.age = 30
This creates a new Person object called john and sets the name and age attributes.
Initializing and Modifying Python Objects
Lets move on to actually initializing Python objects so that you can use them for further coding as well as modifying them as and when needed.
We can initialize the attributes of a Python object using the __init__ method. This method is called when the object is created and allows us to set the initial values of the object’s attributes. For example, let’s modify the Person class to include an __init__ method:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Now, when we create a new Person object, we need to compulsarily provide the name and age parameters as below:
john = Person("John", 30)
We can also modify the attributes of a Python object using ‘dot’ notation. For example, let’s modify the age attribute of the john object:
john.age = 31
This modifies the age attribute of the john object from 30 to 31. So, how about it? Easy Peasy Lemon Squeezy right!
Must-Know Python Class Concepts
Let’s move onto certain important Python Class concepts that you must know and will be a boon to your Python learning journey.
1)Inheritance in Python Classes
Let’s move onto certain important Python Class concepts that you must know and will be a boon to your Python learning journey.
Inheritance is a powerful feature of object-oriented programming that allows classes to inherit attributes and methods from other classes. So, if you define certain methods and attributes in a class, you can use them in a different class without having to define them again! Great right? This makes coding so much easier…
In Python, we can create a new class that inherits from an existing class by including the name of the existing class in parentheses after the new class name. For example, let’s create a new class called Student that inherits from the Person class we created earlier:
class Student(Person):
pass
Here, we have created a new class called Student that inherits from the Person class. The pass statement indicates that we are not adding any new attributes or methods to the Student class.
We can now create instances of the Student class just like we would with the Person class:
student = Student()
student.name = "Alice"
student.age = 20
student.speak("Good morning")
This will create a new Student object, set the name and age attributes, and call the speak method with the greeting “Good morning”.
Since the Student class inherits from the Person class, it also has access to the name and age attributes and the speak method.
2)Polymorphism in Python Classes
Polymorphism is another powerful feature of object-oriented programming that allows objects of different classes to be used interchangeably. In Python, we can achieve polymorphism by using inheritance and overriding methods.
For example, let’s override the speak method in the Student class (since we’ve already covered inheritance) to add a new parameter called subject:
class Student(Person):
def speak(self, greeting, subject):
print(f"{greeting}, my name is {self.name} and I am a {subject} student.")
Now, when we call the speak method on a Student object, we need to provide both the greeting and subject parameters:
student = Student()
student.name = "Alice"
student.age = 20
student.speak("Good morning", "math")
This will print out “Good morning, my name is Alice and I am a math student.” Here, we have used polymorphism to override the speak method in the Student class to add a new parameter.
Encapsulation in Python Objects
Encapsulation in Python is a mechanism that allows data and methods to be hidden from the outside world, preventing unauthorized access and modification. It helps in ensuring data integrity, security, and flexibility of code.
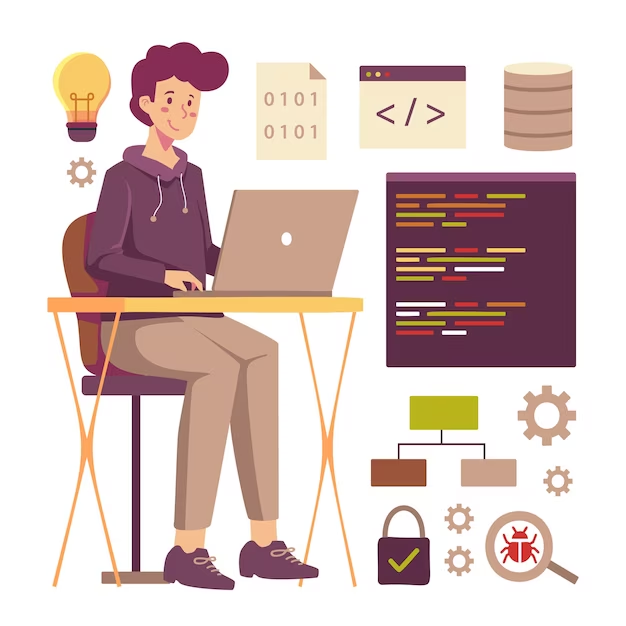
Encapsulation enables developers to create robust, reusable code and simplifies maintenance and debugging, making it an essential feature of Object-Oriented Programming.
In Python, we can achieve encapsulation by using private and protected attributes as well as methods.
1)Private attributes and methods
They are denoted by a double underscore (__) prefix. These attributes and methods cannot be accessed from outside the class. For example, let’s modify the Person class to include a private attribute called __password:
class Person:
def __init__(self, name, age, password):
self.name = name
self.age = age
self.__password = password
Here, we have added a private attribute called __password that cannot be accessed from outside the class.
2)Protected attributes and methods
Denoted by a single underscore (_) prefix, these attributes and methods can be accessed from outside the class, but conventionally should not be modified directly. For example, let’s modify the Person class to include a protected attribute called _email:
class Person:
def __init__(self, name, age, password, email):
self.name = name
self.age = age
self.__password = password
self._email = email
Here, we have added a protected attribute called _email that can be accessed from outside the class, but should not be modified directly.
Top Use Cases for Python Objects
Python objects are incredibly versatile and can be used for a wide variety of applications. Some of them include:
- Creating data models for databases
- Implementing algorithms and data structures
- Building graphical user interfaces (GUIs)
- Developing web applications and APIs
- Building machine learning models
Python Objects greatly simplify these complex development processes.
Best Practices for Creating and Implementing Python Objects
When creating and implementing Python objects, there are a number of best practices to keep in mind:
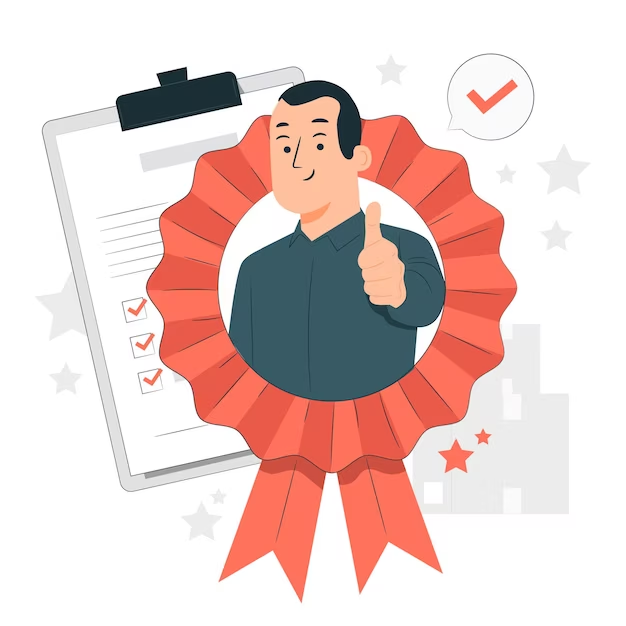
- Use clear and descriptive naming conventions for classes, attributes, and methods.
- Keep classes small and focused on a single responsibility.
- Choose the right level of encapsulation for your attributes and methods.
- Use inheritance and polymorphism to reuse code and build more complex applications.
- Write clear and concise documentation for your classes and methods.
Implementing Python Objects in Real-Life Projects
Python objects are an incredibly powerful tool for building real-life projects. For example, we can use Python objects to build a simple calculator application. Here’s how we can create a Calculator class with three methods, add, subtract, and multiply:
class Calculator:
def add(self, a, b):
return a + b
def subtract(self, a, b):
return a - b
def multiply(self, a, b):
return a * b
Now, we can create a new Calculator object and call its methods:
calculator = Calculator()
print(calculator.add(1, 2)) # Output: 3
print(calculator.subtract(5, 3)) # Output: 2
print(calculator.multiply(4, 6)) # Output: 24
This creates a new Calculator object and calls its add, subtract, and multiply methods with different arguments. This is a very simple application, but you can either build on this code and make a more complex calculator with a range of functions or take up a completely new project!
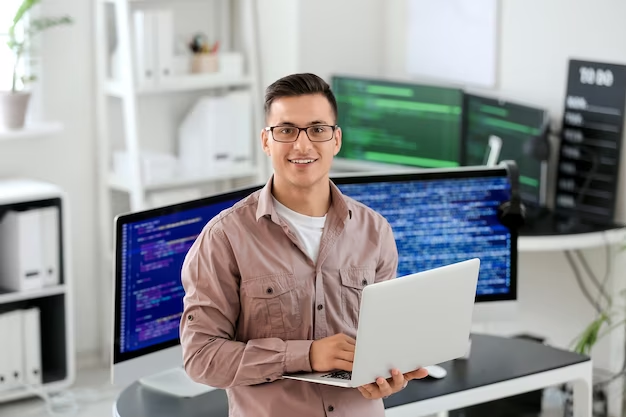
Building projects will not just help you learn but also improve your employability drastically. Click here to get to our blog, where we discuss some fantastic project ideas for you to develop and give your career the boost it needs.
Kickstart your Programming journey by enrolling in GUVI’s Python Career Program where you will master technologies like multiple exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Alternatively, if you would like to explore Python through a Self-Paced course, try GUVI’s Python Self-Paced course.
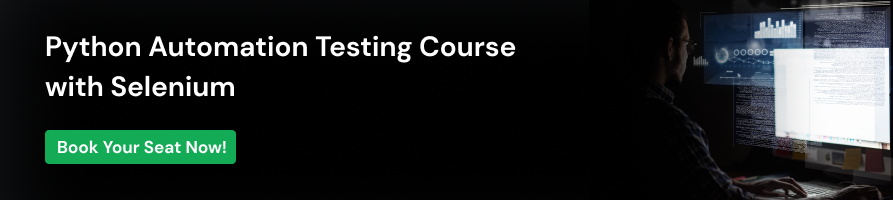
Concluding Thoughts…
In conclusion, Python objects are an essential tool for modern programming. They allow us to create complex and sophisticated applications with ease, while also promoting modularity, code reuse, and maintainability.
Did you enjoy this article?