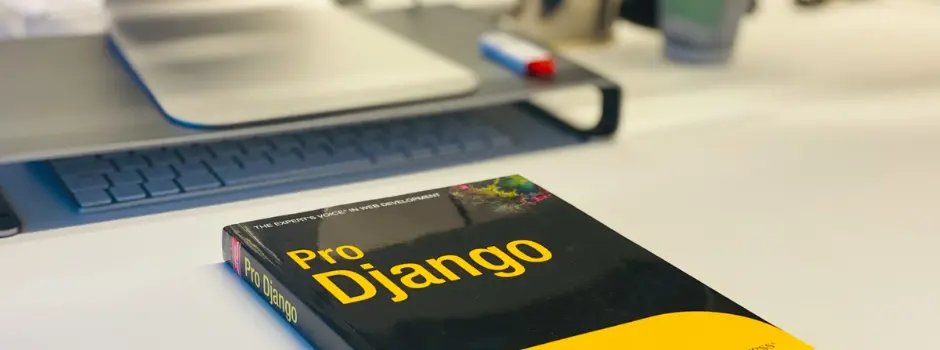
Mastering Django Signals: A Comprehensive Guide [2024]
Apr 24, 2024 7 Min Read 357 Views
(Last Updated)
Django signals, a key feature of the Django framework, empower developers to send automatic notifications, update caches, or perform various tasks seamlessly within a Django application.
By creating ‘hooks’ that activate on specific events, like the creation or deletion of an object, Django signals effectively decouple the action’s sender from its receiver, enhancing code maintainability and reuse.
For those delving into the technical depths of Django, understanding the ins and outs of Django signals will reveal how they facilitate actions such as sending emails upon new account creation or updating search indexes with new content publication.
Covering a gamut from basic implementation to creating and connecting custom signals with receivers, this comprehensive guide aims to equip you with the knowledge to leverage Django signals effectively.
You’ll learn the best practices for working with these powerful mechanisms, ensuring your application’s code is not just efficient but also clean and highly maintainable.
Table of contents
- What Are Django Signals?
- Basics of Implementing Signals in Django
- Creating Custom Signals
- Connecting Signals with Receivers
- Setting Up Your Django Project
- Utilizing Built-in Signals in Django
- Practical Examples of Signal Usage
- Real-world Examples of Django Signals
- Concluding Thoughts...
- FAQs
- What are two important parameters in signals in Django?
- Are Django signals asynchronous?
- How many types of signals are there in Django?
- What is ORM in Django?
What Are Django Signals?
Django signals are essentially a framework feature allowing developers to hook into specific moments in the lifecycle of a model or other Django components and trigger custom code.
These signals are based on the Observer Design Pattern, ensuring a high degree of decoupling between the event producers (senders) and consumers (receivers).
This mechanism is crucial for maintaining clean and modular code in larger applications. Here’s a breakdown of the core aspects of Django signals:
- Types of Signals:
pre_save
/post_save
: Triggered before or after a model instance is saved.pre_delete
/post_delete
: Activated before or after an instance is deleted.pre_init
/post_init
: Fired before or after a model’s__init__
method is called.
- Signal Dispatchers:
- Senders: Components that dispatch signals.
- Receivers: Functions or methods subscribed to signals.
- Connection: Achieved through signal dispatchers, linking senders with their respective receivers.
- Usage Considerations:
- Efficiency: Enables efficient event handling and inter-app communication.
- Modularity: Facilitates decoupling, making applications more modular.
- Sparingly: Should be used judiciously to avoid creating hard-to-debug code.
By adhering to these principles, Django signals offer a powerful method for event-driven programming within Django applications, enhancing both functionality and maintainability.
Also Explore: Top 10 Reasons Why Python is Super Popular in 2024
Before diving into the next section, ensure you’re solid on Python essentials from basics to advanced level. If you are looking for a detailed Python career program, you can join GUVI’s Python Career Program with placement assistance. You will be able to master the Multiple Exceptions, classes, OOPS concepts, dictionary, and many more, and build real-life projects.
Also, if you would like to explore Python through a Self-paced course, try GUVI’s Python Self-Paced course.
Basics of Implementing Signals in Django
To implement Django signals effectively, it’s crucial to understand their components and how they interact within your application. Here’s a simplified breakdown:
- Signal Arguments:
- receiver: The function called when a signal is sent.
- sender: Specifies which sender will trigger the receiver.
- created: A boolean that indicates if a new record has been created.
- instance: The model instance that sent the signal.
- kwargs: Additional data sent with the signal.
- Signal Connection:
- Define a Receiver Function: This function must accept
sender
and**kwargs
as arguments. It contains the logic to be executed when the signal is received. - Connect the Receiver: Use
Signal.connect()
method, specifying the receiver, sender, and optionallyweak
anddispatch_uid
parameters. Thisdispatch_uid
helps prevent duplicate signals. - Sending Signals: Signals can be sent synchronously or asynchronously, allowing for flexible handling. Use
Signal.send()
orSignal.send_async()
methods.
- Define a Receiver Function: This function must accept
- Best Practices:
- Separation of Concerns: Place signals in a
signals.py
module within your app and import them into theAppConfig.ready()
method. - Use Built-in Signals: Leverage Django’s built-in signals like
post_save
for common model events. - Custom Signals: For specific needs, define custom signals using the
Signal
class.
- Separation of Concerns: Place signals in a
By following these guidelines, you can harness the power of Django signals to create dynamic, responsive applications.
Remember, signals are a tool for decoupling components, so use them judiciously to keep your codebase clean and maintainable.
Must Read: Top 7 Must-Know Django Packages
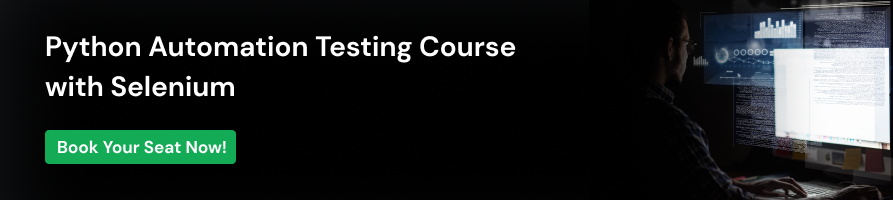
Creating Custom Signals
Creating custom signals in Django allows you to define specific events that your application can respond to.
These custom events can be triggered by various actions within your application, providing a flexible way to implement custom logic. Here’s how you can create and use custom signals:
- Defining Custom Signals: Create a
signals.py
file in your project or app folder. Define your custom signals using theSignal
class. For example:
from django.dispatch import Signal user_logged_in = Signal(providing_args=["request", "user"])
This code snippet creates a custom signal named user_logged_in
that expects request
and user
arguments.
Also Read About Python Objects 101: How to Create and Master Them With Real-World Projects
- Creating Receivers:
- In the
receivers.py
file within the same folder, define functions that will act as receivers for your custom signals. Example:
from django.dispatch import receiver
from .signals import user_logged_in
@receiver(user_logged_in)
def handle_user_login(sender, **kwargs):
# Custom logic here
- Register your receivers in the
apps.py
file of your app by overriding theready
method:
from django.apps import AppConfig
class YourAppConfig(AppConfig):
name = 'your_app'
def ready(self):
import your_app.signals
- Triggering Signals:
- Signals can be sent from views or any other place in your code. For example, to trigger the
user_logged_in
signal when a user logs in:
from .signals import user_logged_in
def your_view(request):
# Your login logic here
user_logged_in.send(sender=self.__class__, request=request, user=user)
- This sends the
user_logged_in
signal with the appropriate arguments, which can then be caught and handled by the registered receiver.
- By following these steps, you can create custom signals to enforce business rules or trigger actions based on specific conditions in your Django application.
Also Read: 6 Essential Python Modules: A Comprehensive Guide
Connecting Signals with Receivers
To effectively connect Django signals with receivers, follow these technical steps to ensure your application responds dynamically to specific events:
- Signal Registration:
- Place your signals in a dedicated
signals.py
file within your app’s directory to maintain a clean code structure.
- In your app’s
apps.py
file, import the signals module inside theAppConfig.ready()
method. This guarantees that your signals are registered when the Django application starts up. Example:
from django.apps import AppConfig
class MyAppConfig(AppConfig):
name = 'my_app'
def ready(self):
import my_app.signals
Also, Find Out What Does the yield Keyword In Python Do?
- Connecting Signals to Receivers:
- Use the
Signal.connect()
method to link a signal to its receiver function. This connection can be made directly in thesignals.py
file or within theAppConfig.ready()
method for application-wide signals. Example:
from django.db.models.signals import post_save
from django.dispatch import receiver
from my_app.models import MyModel
from my_app.signals import my_custom_signal
@receiver(post_save, sender=MyModel)
def my_model_post_save(sender, instance, created, **kwargs):
if created:
my_custom_signal.send(sender=instance.__class__, instance=instance)
- Ensure each receiver function accepts a
sender
parameter and wildcard keyword arguments (**kwargs
). Utilize thedispatch_uid
parameter to avoid duplicate signals.
- Signal Disconnection:
- To uncouple a receiver from a signal, utilize the
Signal.disconnect()
method, providing the same parameters used during connection. This is particularly useful for temporary listeners or cleaning up in tests.
- By adhering to these steps, you’ll efficiently connect signals with receivers in your Django project, enabling responsive and modular applications. Remember, signals should be used judiciously to maintain code clarity and prevent unnecessary complexity.
Must Explore: Python * Single And **Double Asterisk Explained!
Setting Up Your Django Project
Setting up your Django project to effectively use Django signals involves a few critical steps that ensure your application can respond to events seamlessly. Given the technical nature of this setup, let’s dive into the specifics:
- Organize Your Signals:
- Create a
signals.py
Module: For each Django app in your project, create a separatesignals.py
file. This module will house all your signal definitions, making them easy to find and manage. Example:
# In your_app/signals.py
from django.dispatch import Signal
# Define your custom signals here
user_registered = Signal(providing_args=["user", "request"])
Must Explore: How To Use Global Variables Inside A Function In Python?
- AppConfig and Signal Registration:
- Modify
apps.py
: In the correspondingapps.py
file of your app, ensure you have an AppConfig class defined. Within this class, override theready
method to import thesignals.py
module. This step is crucial as it connects your signals with Django’s application loading mechanism. Example:
# In your_app/apps.py
from django.apps import AppConfig
class YourAppConfig(AppConfig):
name = 'your_app'
def ready(self):
# Import signals to ensure they're registered
import your_app.signals
- Update
__init__.py
:
- AppConfig Reference: Make sure your application’s
__init__.py
file points to the AppConfig class you modified. This ensures Django uses the configuration where your signals are registered upon startup. Example:
# In your_app/__init__.py
default_app_config = 'your_app.apps.YourAppConfig'
By following these steps, your Django project will be primed to utilize signals, allowing for a modular and responsive application architecture. This setup ensures that your signals are neatly organized and automatically registered with Django, facilitating smooth operation within your project.
Read: Python | List Of Lists Changes Reflected Across Sublists
Utilizing Built-in Signals in Django
Django’s built-in signals provide a robust framework for responding to various events throughout the lifecycle of both model instances and the request/response cycle.
Understanding and utilizing these signals effectively can significantly enhance the functionality and responsiveness of your Django applications. Here’s a breakdown of the key built-in signals and how they can be employed:
- Model Signals:
pre_save
andpost_save
: Trigger actions before or after a model instance is saved. Use these to manipulate data before it’s committed to the database or to trigger actions after saving.pre_delete
andpost_delete
: Implement logic to execute before or after an instance is deleted, such as cleaning up related data or sending notifications.pre_init
andpost_init
: Useful for setting up or modifying instance attributes directly before or after the model’s__init__
method is called.
- Request/Response Signals:
- These signals are invaluable for executing code at different stages of the request/response cycle. For instance, logging or modifying the request before it reaches the view or altering the response before it’s sent to the client.
- Signal Sending Methods:
- Django allows signals to be sent synchronously (
Signal.send()
,Signal.send_robust()
) or asynchronously (await Signal.asend()
,await Signal.asend_robust()
), providing flexibility in how and when signal receivers are executed. This feature is particularly useful for operations that can be performed outside the immediate flow of execution, such as sending emails or processing data in the background.
- Django allows signals to be sent synchronously (
Leveraging these built-in signals enables developers to create more dynamic, efficient, and maintainable Django applications by decoupling event-handling logic from the core application code.
Do Read: Popular 6 Python Libraries for Parallel Processing
Practical Examples of Signal Usage
In the realm of Django development, signals offer a powerful mechanism for executing code in response to certain actions or events. Here are practical examples of how signals can be utilized effectively in your Django projects:
- Automatic User Profile Creation:
- Models.py: Define a
UserProfile
model that will store additional information about your users. - Signals.py:
from django.db.models.signals import post_save
from django.dispatch import receiver
from django.contrib.auth.models import User
from .models import UserProfile
@receiver(post_save, sender=User)
def create_user_profile(sender, instance, created, **kwargs):
if created:
UserProfile.objects.create(user=instance)
This signal listens for the post_save
event of the User
model. If a new user is created (created=True
), a corresponding UserProfile
instance is also created automatically.
- Triggering Email Notifications:
- Signals.py:
from django.core.mail import send_mail
from django.dispatch import receiver
from .signals import user_registered
@receiver(user_registered)
def send_welcome_email(sender, **kwargs):
user = kwargs.get('user')
send_mail(
'Welcome to Our Site',
'Thank you for registering.',
'[email protected]',
[user.email],
fail_silently=False,
)
Whenever the user_registered
signal is sent, this receiver function triggers an email to the newly registered user, welcoming them to the site.
- API Class Triggering: Suppose you have two API views where the completion of one triggers the start of another. Using signals, you can decouple these views by sending a signal at the end of the first view’s request that the second view listens for, enabling a seamless transition between processes without tightly coupling the views’ code.
These examples showcase the versatility of Django signals in automating tasks, enhancing user experience, and maintaining a clean separation of concerns within your application code.
To Learn: 7 Best Websites to Learn Python for Free
Real-world Examples of Django Signals
Django signals play a pivotal role in building responsive, efficient applications by reacting to changes in your models or system. Here are some real-world examples showcasing the versatility and power of Django signals:
- User Engagement and Management:
- Automatically create a user profile with the
post_save
signal upon user registration, enhancing the user experience without cluttering the registration logic. - Send personalized email notifications using the
post_save
signal when users receive new comments or updates, fostering community engagement. - Implement a system to automatically deactivate users after 90 days of inactivity using the
pre_save
signal, maintaining an active user base.
- Automatically create a user profile with the
- Data Integrity and Synchronization:
- Maintain an audit trail by logging changes to critical records with the
post_save
signal, ensuring data transparency and accountability. - Use the
post_delete
andpost_save
signals to invalidate cached data, ensuring users always receive up-to-date information. - Synchronize data with external systems or APIs seamlessly with the
post_save
signal, keeping your application’s data consistent across platforms.
- Maintain an audit trail by logging changes to critical records with the
- Security and Compliance:
- Dynamically adjust user permissions based on specific actions or conditions using the
post_save
signal, enhancing application security. - Implement checks with the
pre_delete
signal to maintain database integrity and prevent accidental data loss.
- Dynamically adjust user permissions based on specific actions or conditions using the
These examples illustrate how Django signals can be employed to automate tasks, enhance user interaction, and maintain a clean, decoupled architecture in your Django project.
Also, Find Out if you Know How To Create Variables In Python?
Kickstart your Programming journey by enrolling in GUVI’s Python Career Program where you will master technologies like multiple exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Alternatively, if you would like to explore Python through a Self-Paced course, try GUVI’s Python Self-Paced course.
Concluding Thoughts…
Throughout this comprehensive guide, we have navigated the intricate landscape of Django signals, unveiling their powerful utility in enhancing application responsiveness and maintainability.
As we conclude, it’s paramount to reflect on the broader implications of employing Django signals within your projects.
Beyond the immediate benefits of code efficiency and maintainability, leveraging signals effectively prepares the ground for scalable, modular applications that can adapt and thrive amidst evolving technological landscapes.
Also Read: Top 6 Backend Frameworks That You Should Know in 2024
FAQs
What are two important parameters in signals in Django?
In Django signals, two important parameters are sender
and kwargs
. Read the article above to learn more about them.
Are Django signals asynchronous?
No, Django signals are synchronous.
How many types of signals are there in Django?
There are two types of signals in Django: pre_save
and post_save
.
Did you enjoy this article?