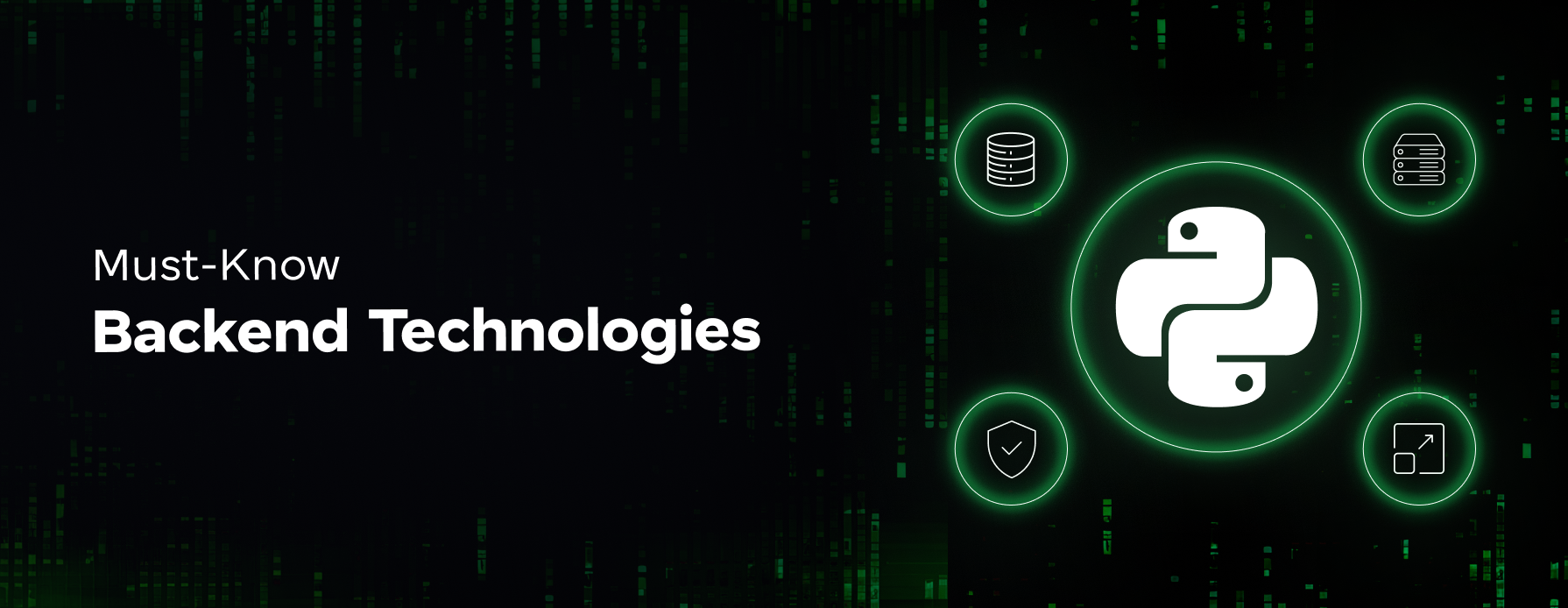
Becoming A Top Python Backend Developer: Must-Know Technologies [2024]
Apr 30, 2024 10 Min Read 380 Views
(Last Updated)
Python, a versatile and dynamic programming language, stands out in the realm of backend development for its simplicity and efficiency.
As the demand for skilled Python backend developers continues to surge, those adept in this language find themselves among the most sought-after and well-compensated professionals, particularly due to Python’s critical role in enhancing user experiences and automating tasks.
This article delves into the essential technologies that Python backend developers must master to excel in their field.
Each technology presents an opportunity to elevate your backend development skills, making this guide an indispensable resource for professionals looking to refine their expertise and advance in their careers.
Table of contents
- Understanding Python's Role in Back-End Development
- Core Technologies for Python Backend Developers
- 1) Django
- 2) Flask
- 3) Docker and Kubernetes
- 4) FastAPI
- 5) Tornado and Its Use Cases
- 6) Pyramid
- 7) SQLAlchemy
- 8) Celery for Asynchronous Task Queues
- 9) Redis
- Conclusion
- FAQs
- Which technology is used for backend?
- What are the 3 languages used by backend programmers?
- Is Python a backend language?
- Is Python fast for backend?
Understanding Python’s Role in Back-End Development
Familiarity with Python is not just beneficial but crucial for anyone looking to excel in backend development. This stems from several key factors that make Python a top choice for developers around the globe:
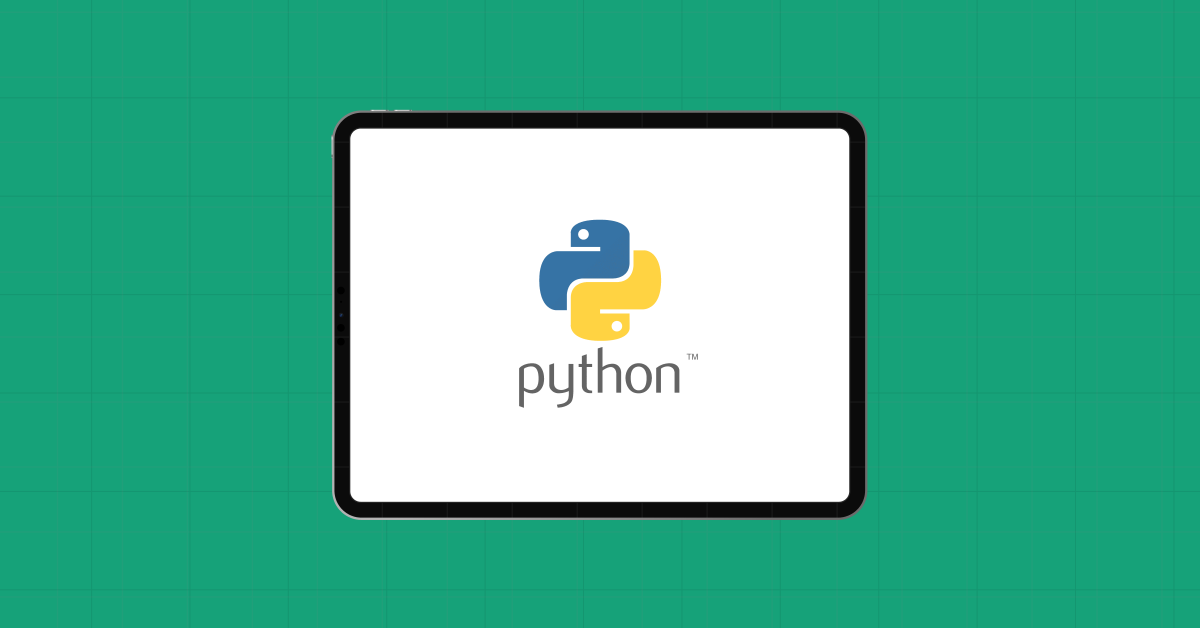
- Ease of Learning: Python’s straightforward syntax mirrors everyday English, which significantly lowers the barrier to entry for beginners. Its simplicity enables both novices and seasoned professionals to pick up the language quickly, making Python one of the easiest programming languages to learn.
- High Demand: The demand for Python developers is soaring, with industry giants like Google, Netflix, Spotify, and Pinterest relying on Python for their backend services. This widespread adoption underscores Python’s utility and efficiency in handling back-end tasks.
- Versatility: Python’s application is vast, ranging from web development and data processing to more advanced fields like machine learning, cybersecurity, and even 3D modeling. This versatility allows developers to apply their Python skills across various domains, enhancing their value in the job market.
Python’s popularity and efficacy in backend development are further bolstered by its mature ecosystem:
- Robust Frameworks and Tools: Python boasts a rich collection of frameworks and development tools, such as Django and Flask for web development, and pip for package management. These tools streamline the development process, improving productivity and code quality.
- Vibrant Community: With one of the largest active communities, Python developers have access to a wealth of resources and quick problem resolution. This community support is vital for continuous learning and keeping abreast of the latest developments in Python backend development.
- Clean Syntax and Readability: Python’s clean syntax promotes readability and ease of maintenance, making it easier for developers to collaborate on projects. This attribute is particularly beneficial in complex backend systems where clarity and maintainability are paramount.
In essence, Python serves as a universal language suitable for a wide range of applications. Its simplicity, readability, and vast ecosystem of libraries empower developers to build robust and scalable web applications efficiently.
Whether you’re just starting your journey in backend development or looking to enhance your existing skills, Python offers a solid foundation and a plethora of growth opportunities.
Before diving into the next section, ensure you’re solid on Python essentials from basics to advanced level. If you are looking for a detailed Python career program, you can join GUVI’s Python Career Program with placement assistance. You will be able to master the Multiple Exceptions, classes, OOPS concepts, dictionary, and many more, and build real-life projects.
Also, if you would like to explore Python through a Self-paced course, try GUVI’s Python Self-Paced course.
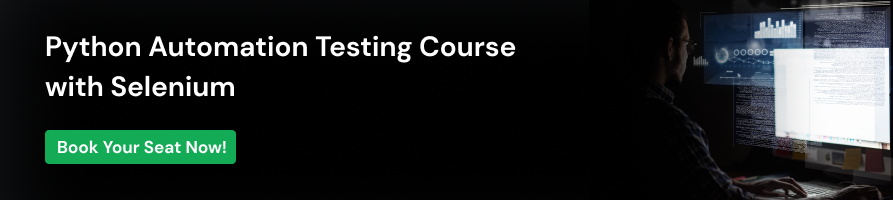
Core Technologies for Python Backend Developers
To excel as a Python backend developer, mastering a variety of technologies and frameworks is essential. Here’s a breakdown of the core technologies you should focus on:
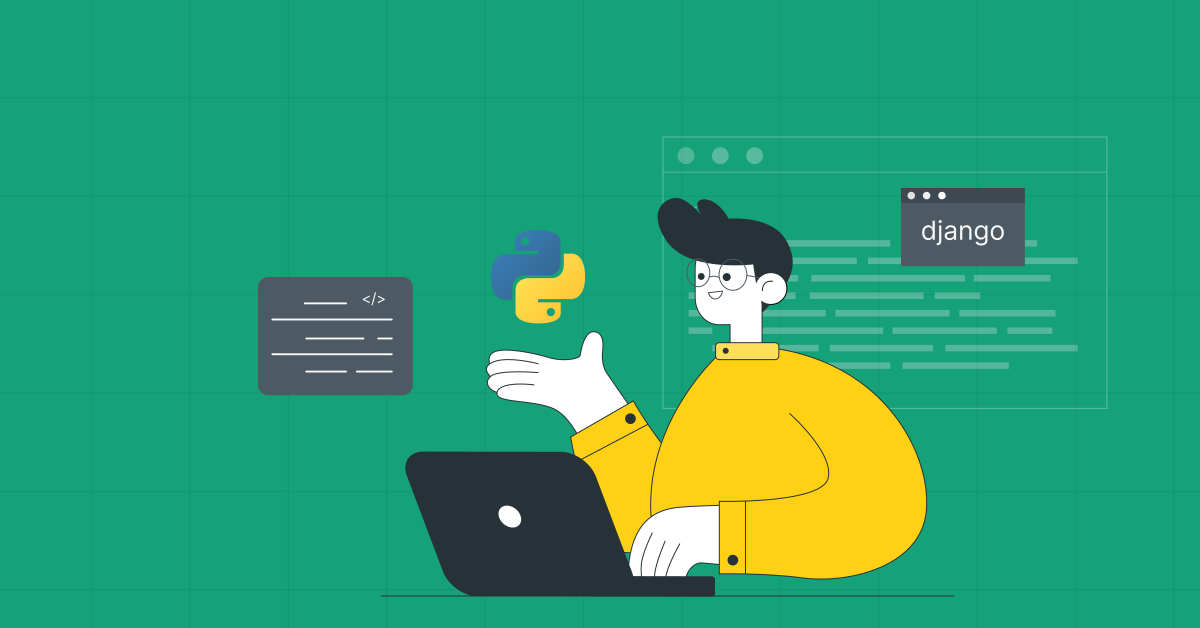
- Python Frameworks:
- Flask: A lightweight WSGI web application framework. It’s easy to get started with and is suitable for small to medium projects that require a solid web application without much overhead.
- Django: Known for its “batteries-included” approach, Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It’s ideal for developers looking to build robust web applications with less code.
- FastAPI: An asynchronous framework that is gaining popularity for its high performance. It’s designed to build APIs with Python 3.6+ based on standard Python-type hints.
- Tornado: A Python web framework and asynchronous networking library, Tornado is particularly well-suited for long polling, WebSockets, and other applications that require a long-lived connection to each user.
- Pyramid: A flexible, modular framework that lets you choose your database, URL structure, templating style, and more. It’s ideal for projects that need to scale or evolve.
- Key Concepts and Tools:
- Python Programming Fundamentals: Understand syntax, data types, control flow, functions, and object-oriented programming (OOP) concepts.
- Web Development Basics: Gain knowledge in HTML, CSS, and JavaScript to understand how the frontend interacts with your Python backend.
- Database Management: Learn SQL for relational databases like MySQL or PostgreSQL, and explore NoSQL databases like MongoDB or Redis for different data storage needs.
- API Development: Design and build APIs using frameworks like Flask or Django REST framework to enable communication between different software applications.
- Version Control: Master Git, a version control system widely used in software development, to track changes and collaborate with other developers.
- Testing and Debugging: Develop proficiency in writing tests for your code and learn debugging and troubleshooting techniques to ensure your applications run smoothly.
- Security and Authentication: Understand web security basics, protect against common vulnerabilities like XSS and SQL injection attacks, and implement authentication and authorization mechanisms.
By focusing on these technologies and following the outlined steps, you’ll be well on your way to excelling in Python backend development. Now, we shall explore some of these and more in detail.
1) Django
Django stands as a beacon for Python backend developers seeking a comprehensive framework that simplifies the development process while maintaining high performance and security.
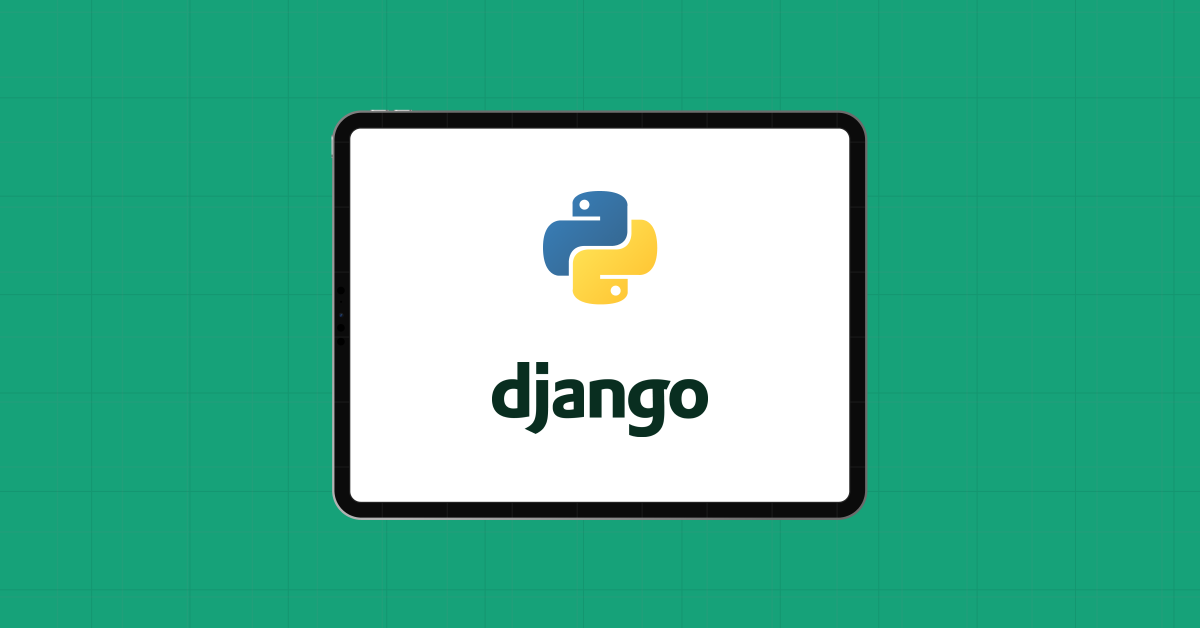
Its architecture, inspired by the MVC (Model-View-Controller) pattern, is designed to facilitate rapid development and pragmatic design. Here’s a deeper dive into the core components and features that make Django a top choice for backend development:
- Object-Relational Mapper (ORM):
- Simplifies database interactions by allowing developers to use Python code instead of SQL queries.
- Enhances code maintainability and application scalability.
- Automatic Admin Interface:
- Generates a ready-to-use administrative interface for managing application data.
- Reduces development time by eliminating the need to manually create CRUD interfaces.
- Templating System:
- Separates presentation from business logic, enabling developers to create dynamic web pages with ease.
- Supports inheritance and reusable templates, promoting DRY (Don’t Repeat Yourself) principles.
- URL Routing:
- Offers a clean and elegant URL scheme that is easy to manage and scale.
- Facilitates the creation of SEO-friendly URLs.
Django REST Framework (DRF) extends Django’s capabilities, providing a powerful toolkit for building RESTful APIs. Key features include:
- Serialization: Converts complex data types into JSON, XML, or other content types for easy frontend consumption.
- Authentication and Permissions: Offers a flexible system for managing user access and permissions.
- Pagination and Filtering: Simplifies the handling of large datasets by implementing pagination and custom data filtering.
Building a RESTful API with Django and DRF involves a straightforward process:
- Setup: Install Django and DRF.
- Project and App Creation: Initialize a new Django project and create a Django app.
- Model Definition: Design your data model (e.g., a
Book
model with fields liketitle
,author
, andpublication_date
). - Serialization: Use DRF’s serializers to define how your data model translates to JSON.
- View Creation: Implement views to handle CRUD operations on your model.
- URL Routing: Configure URL patterns to map requests to the appropriate views.
- Testing: Ensure your API works as expected by performing thorough tests.
Django’s philosophy of “Don’t Repeat Yourself” ensures that developers can focus on writing unique application logic rather than reinventing the wheel for each new project.
Its robust security features, including protection against SQL injection, cross-site scripting, and clickjacking, make it a trusted choice for developers worldwide.
Whether building a simple website or a complex, high-load web application, Django’s flexibility, reliability, and scalability make it an invaluable tool in a Python backend developer’s arsenal.
2) Flask
Flask emerges as a standout choice for Python backend development, especially due to its microframework nature which is built on the WSGI toolkit and Jinja2 template engine.
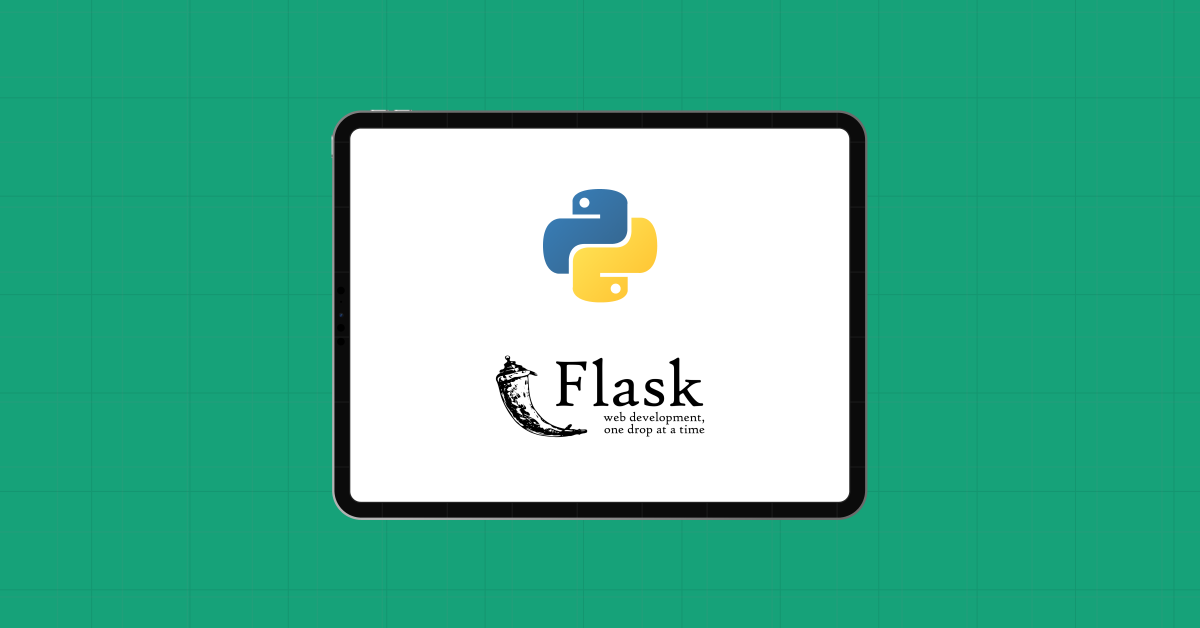
This foundation not only makes Flask lightweight but also incredibly flexible and efficient for developers. Here’s a closer look at what sets Flask apart:
- Minimalist Approach: Flask’s design philosophy is centered around being as minimalistic as possible. This means it comes with only the essentials needed to get a web application off the ground, leaving the rest up to you. This approach allows you to add only what you need through extensions and libraries, keeping your project lightweight and manageable.
- Versatility and Scalability: Despite its simplicity, Flask does not compromise on functionality. It’s perfectly suited for both small projects and large-scale applications, thanks to its scalability. Whether you’re building a simple web application or a complex RESTful API, Flask provides the tools necessary to meet your project’s requirements.
- Rapid Development and Debugging: Flask includes a built-in development server and a fast debugger. This setup is invaluable for developers, as it facilitates quick iterations and immediate feedback on changes. It significantly reduces the time spent on identifying and resolving issues, making the development process more efficient.
Flask’s compatibility with various web servers and platforms further enhances its appeal. Whether deploying to Heroku, Google App Engine, or AWS Elastic Beanstalk, Flask ensures your application can be easily adapted to different environments. This wide range of compatibility is crucial for developers looking to maintain flexibility in their deployment options.
Key Features and Considerations:
- RESTful Request Dispatching: Supports the creation of RESTful APIs, making it an ideal choice for backend services that require efficient communication with the frontend.
- Built-in Development Tools: The integrated support for unit testing, alongside the development server and debugger, ensures that applications are robust and error-free.
- Creative Control: Flask’s templating engine provides the freedom to maintain a consistent user interface across multiple pages, enhancing the user experience.
- Security and Modules: While Flask’s extensibility is a significant advantage, it’s important to exercise caution when incorporating third-party modules, as this could introduce security vulnerabilities.
However, it’s worth noting that Flask’s simplicity can be a double-edged sword. In the hands of inexperienced developers, there’s a risk of producing low-quality code.
Additionally, Flask’s default sequential request handling may not be suitable for applications expecting high traffic volumes, potentially leading to delays.
In summary, Flask offers a compelling combination of simplicity, flexibility, and efficiency for Python backend development. Its lightweight nature, coupled with the ability to scale and adapt to various project requirements, makes it an excellent choice for both beginners and seasoned developers.
3) Docker and Kubernetes
In the realm of Python backend development, the deployment and management of applications are significantly streamlined by the adoption of Docker and Kubernetes.
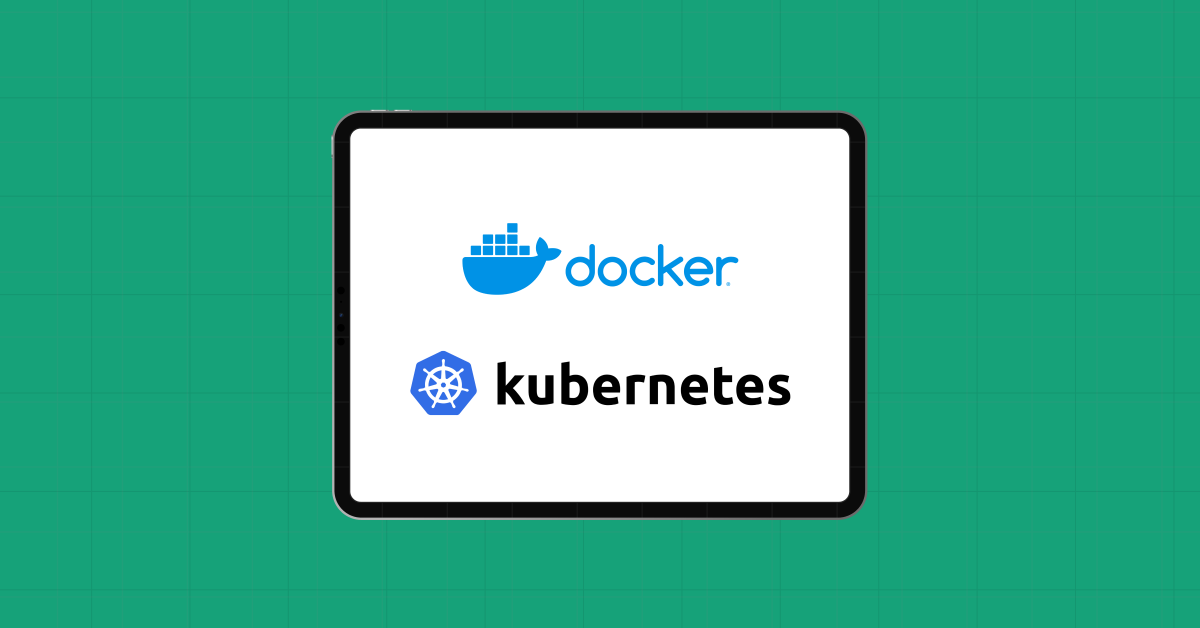
These tools have become indispensable for developers seeking efficiency and consistency across various environments. Here’s a closer look at how Docker and Kubernetes revolutionize backend development:
- Docker: The Containerization Champion
- Portability and Consistency: Docker containers encapsulate an application and its dependencies into a single package, ensuring that it runs uniformly and consistently across any environment. This eliminates the “it works on my machine” problem, a common headache for developers.
- Simplified Deployment: By using Docker, you can easily move your application from development to test environments and finally to production without any changes. Docker containers can be deployed on any system that supports Docker, which includes most modern Linux distributions, macOS, and Windows.
- Docker Hub and Desktop: Docker Hub acts as a registry service, simplifying the sharing and deployment of containers. Docker Desktop, available for Windows, macOS, and Linux, integrates local Kubernetes support, offering a seamless development experience.
- Kubernetes: Master of Container Orchestration
- Automated Scaling and Management: Kubernetes intelligently manages containerized applications across multiple hosts. It automatically handles scaling based on demand, rolling updates, and system failures, ensuring high availability and efficient resource use.
- Powerful Tools for Developers: Kubernetes offers a set of APIs and tools that simplify deploying, scaling, and managing Docker containers. Its compatibility with Docker makes it an excellent choice for developers familiar with Docker.
- Enhanced Backend Development: Features such as auto-restarting, liveness, and readiness probes, and autoscaling are particularly relevant for backend applications. These capabilities ensure that applications are always available and perform optimally under varying loads.
Understanding and mastering Docker and Kubernetes can significantly enhance a Python backend developer’s skill set.
These technologies not only improve the deployment process but also offer robust solutions for managing complex applications at scale.
As the demand for scalable and reliable backend systems grows, familiarity with Docker and Kubernetes will undoubtedly open new doors for career advancement in the competitive field of backend development.
4) FastAPI
FastAPI emerges as a groundbreaking choice for Python backend developers aiming for high performance and efficiency. Here’s a closer look at its standout features:
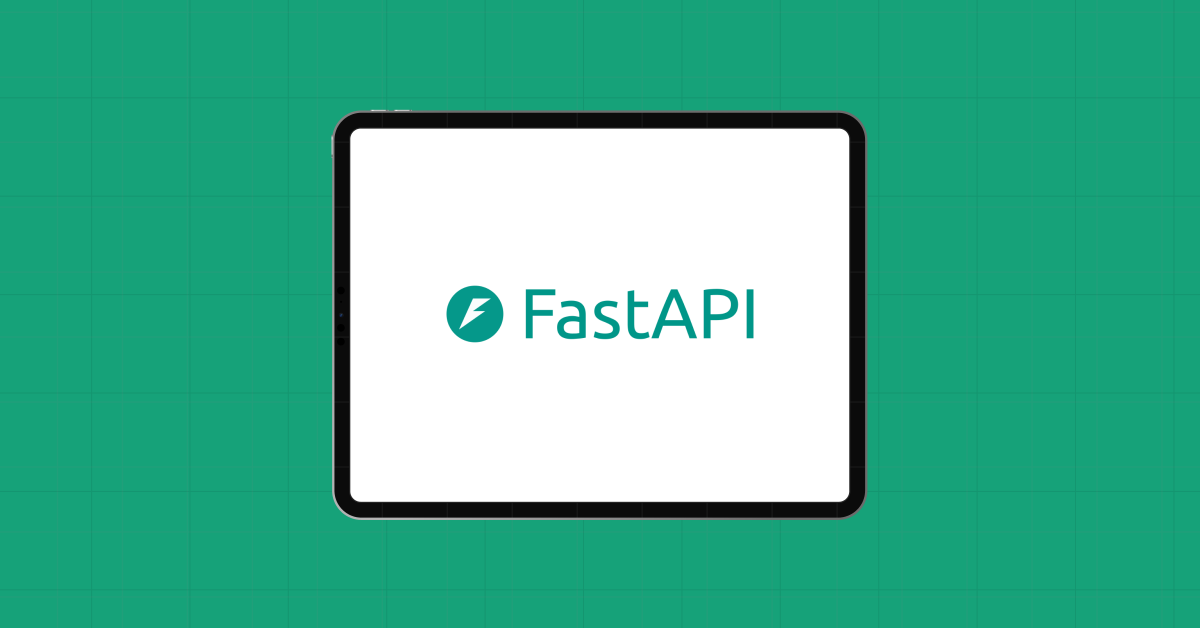
- High Performance:
- Comparable to NodeJS and Go, FastAPI’s speed is a game-changer in the Python ecosystem.
- Its asynchronous request-handling mechanism significantly boosts performance, making it an ideal choice for high-traffic applications.
- Robust Data Validation:
- Built-in data validation ensures that the data your API works with is correct and secure, reducing the risk of errors and vulnerabilities.
- This feature is powered by Pydantic, enhancing code reliability and developer productivity.
- Comprehensive Documentation:
- FastAPI automatically generates interactive API documentation using Swagger UI and ReDoc. This not only aids in testing and debugging but also simplifies the developer experience.
- The documentation is straightforward, providing excellent editor support, which accelerates development cycles.
FastAPI is not just about speed; it’s about making development enjoyable and efficient. With FastAPI, you can expect:
- Reduced Bug Risks: The coding style advocated by FastAPI can lead to a significant reduction in induced bugs, by approximately 40%. This makes application maintenance easier and more reliable.
- Open Standards Compatibility: Embracing open standards like OpenAPI and JSON Schema, FastAPI ensures that your APIs are built on a solid, standardized foundation, facilitating easier integration with other services and tools.
- Adoption by Leading Companies: Its robustness and performance have led companies like Uber and Netflix to use FastAPI for building their applications, which speaks volumes about its reliability and scalability in real-world scenarios.
Moreover, the framework’s foundation on Starlette for the web parts and Pydantic for the data parts provides developers with a powerful yet enjoyable toolset for crafting modern web APIs.
The significant increase in development speed (200% to 300%) and the minimization of code duplication highlight FastAPI’s capacity to streamline backend development processes, making it easier to maintain and scale applications.
Whether you’re a beginner or an experienced developer, FastAPI offers a compelling combination of speed, ease of use, and comprehensive features that can enhance your Python backend projects.
5) Tornado and Its Use Cases
Tornado, a robust Python web framework and asynchronous networking library, offers unique advantages for backend development, particularly in environments demanding high concurrency and low latency. Here’s a technical breakdown of Tornado’s capabilities and use cases:
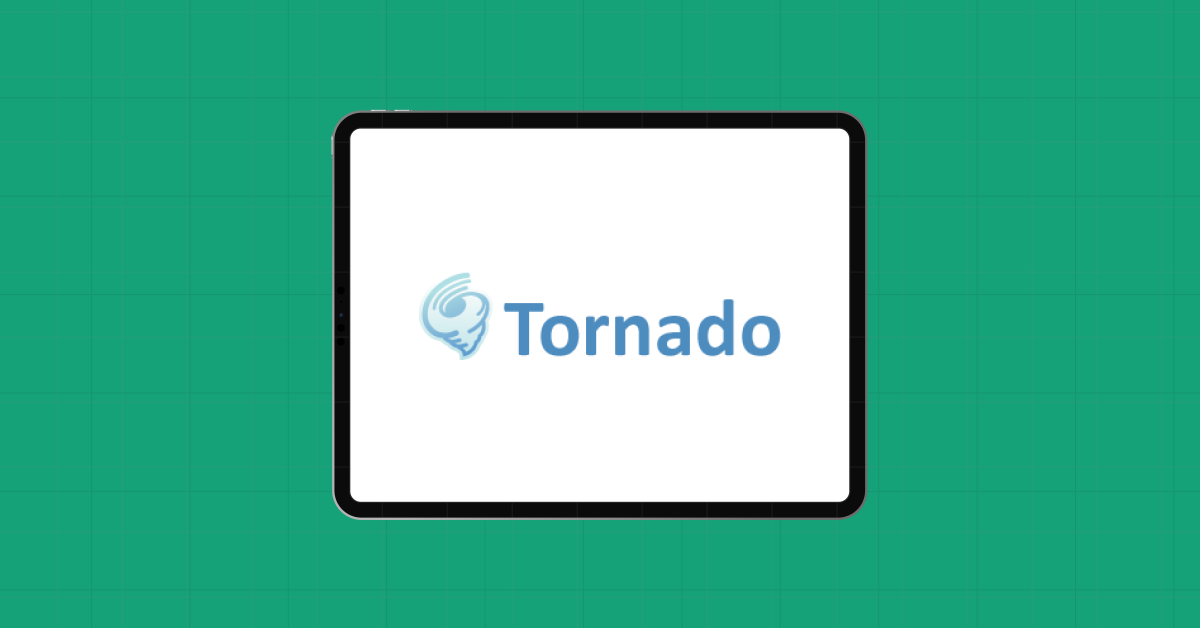
- Asynchronous Capabilities:
- Utilizes non-blocking network I/O to manage thousands of active connections, making it adept at handling applications with a vast number of simultaneous user interactions.
- Integrates with Python’s standard
asyncio
library, allowing for a seamless mixing of Tornado andasyncio
-based libraries within the same event loop for efficient asynchronous programming.
- Performance and Scalability:
- Its event loop design accelerates request execution, enabling Tornado to scale to tens of thousands of open connections, a feature particularly beneficial for real-time applications like chat services, online games, and real-time analytics.
- Not confined by the traditional WSGI, Tornado can bypass some of the limitations associated with synchronous request handling, providing a pathway to build highly responsive applications.
- Development Flexibility:
- Tornado’s
Application
object facilitates straightforward setup for routing, views, and database configurations, streamlining the development process. - Offers class-based views, differing from the function-based views seen in Flask and Pyramid, granting developers a higher degree of control and customization over request handling.
- Despite its lower-level nature, which may necessitate a deeper understanding of routing, templating, and authentication, Tornado remains a powerful tool for developers with a solid grasp of Python and web development fundamentals.
- Tornado’s
Tornado’s specialized focus on applications requiring long-lived connections to each user, such as those utilizing WebSockets or long polling, underscores its position as a go-to framework for projects demanding high performance under concurrent user demands.
While it may not be the first choice for applications with intricate business logic or heavy data processing needs, its efficiency in handling high concurrency and low latency tasks makes it an invaluable asset in the modern developer’s toolkit, especially when used in conjunction with other Python frameworks to manage specific aspects of an application.
6) Pyramid
Pyramid, a Python backend framework, is celebrated for its flexibility and simplicity, making it a prime choice for developers with projects that range from small to ambitious.
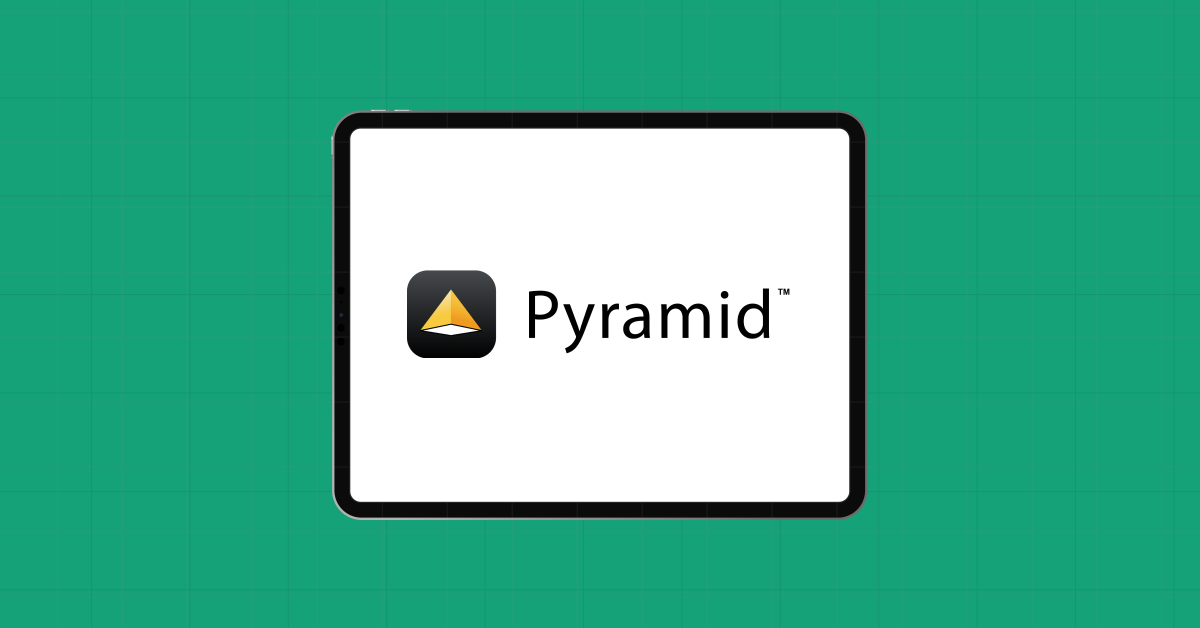
Its design philosophy encourages starting with a minimal setup, which can be expanded with a wide array of add-ons and packages as the project’s needs grow. This approach ensures that developers are not overwhelmed from the outset but can scale their applications efficiently.
Key features include:
- Scalability and Flexibility:
- Start small with a simple “hello world” app and expand as needed.
- A rich ecosystem of add-ons and extensions for customization.
- Control and Quality:
- Avoids framework magic for better control over application behavior.
- Committed to API stability, bug fixing, and comprehensive test and documentation coverage.
Pyramid’s architecture supports a variety of templating engines, form systems, and databases, providing developers with the freedom to choose the best tools for their specific project requirements without being locked into a single approach. This level of decision support is crucial for maintaining high-quality, performance-oriented applications. Pyramid’s core features that support this include:
- Powerful URL Routing System: Intuitive setup for routes with various patterns and parameters.
- Highly Extensible Architecture: Facilitates the integration of third-party tools and libraries.
- Support for Test-Driven Development (TDD): Tools and patterns for straightforward unit testing.
The community around Pyramid is growing, offering a wealth of resources, from extensive narrative documentation and API references to a curated list of applications built with Pyramid.
This community support, combined with the framework’s emphasis on craftsmanship and quality, makes Pyramid a future-proof choice for Python backend development.
Notable users of Pyramid, such as Mozilla and Survey Monkey, underscore its capability to support high-performance web applications across various industries.
7) SQLAlchemy
SQLAlchemy stands as a pivotal library in the Python backend development landscape, bridging the gap between Python programs and various databases.
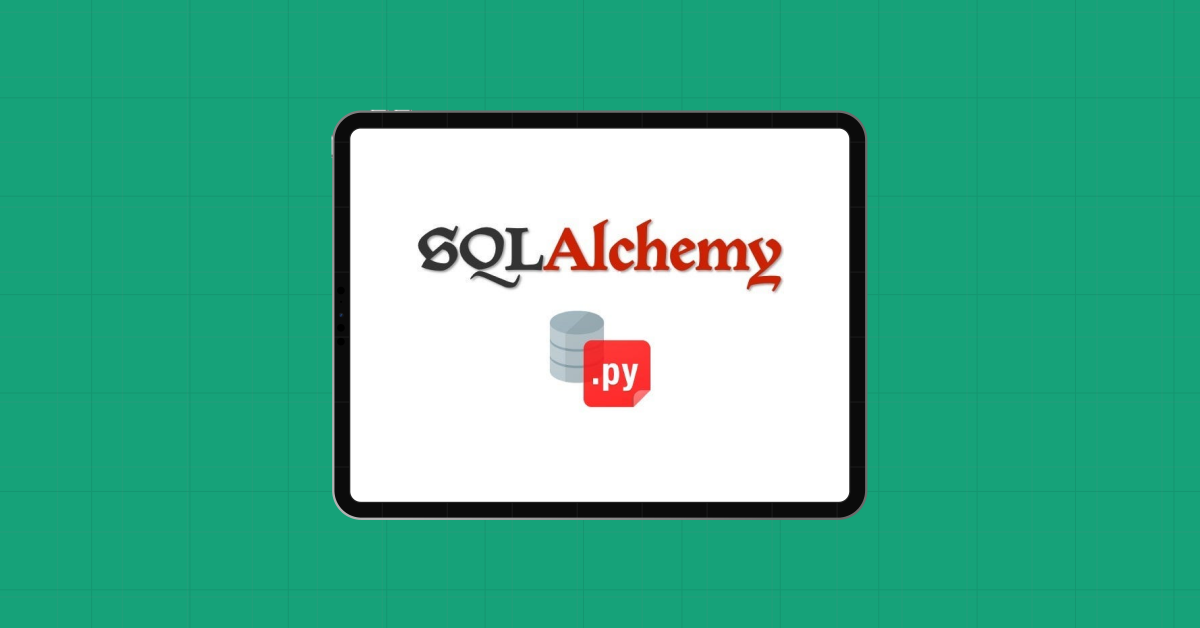
It’s renowned for its Object Relational Mapper (ORM) capabilities, which facilitate an intuitive and efficient way to manage database operations. Here’s a closer look at its core components and functionalities:
- Core Components:
- Engines: Serve as the foundation, managing Pools and Dialects.
- Pools: Optimize performance by caching pre-initialized connections, reducing overhead.
- Dialects: Enable communication with different database engines by handling SQL syntax variations.
- ORM: Specializes in mapping database tables to Python classes, supporting relationships like One To Many and Many To One.
- Sessions: Implement the Unit of Work pattern, ensuring database consistency by applying changes in a coordinated manner.
- Engines: Serve as the foundation, managing Pools and Dialects.
- Key Functionalities:
- Database-Agnostic Code: Allows for the creation of code that’s not tied to a specific database, enhancing portability.
- Data Validation and Constraints: Enforces data integrity by preventing the insertion of invalid data.
- Query Optimization: Facilitates efficient database queries, optimizing application performance.
- CRUD Operations: Simplifies Create, Read, Update, and Delete operations through an object-oriented approach.
- Getting Started with SQLAlchemy:
- Installation: Use
pip install sqlalchemy
to add SQLAlchemy to your project. - Connecting to a Database: Create an engine with
sqlalchemy.create_engine()
, specifying your database’s address. - Reflecting Tables: Automatically load table definitions using reflection, a process that builds metadata from existing database structures.
- Interacting with Data:
- Querying: Utilize methods like
where
,in
, andorder by
for data retrieval. - Inserting and Updating Data: Use
db.insert(table_name).values(data)
anddb.update(table_name).values(attribute=new_value).where(condition)
for data manipulation. - Handling Large ResultSets: Employ
.fetchmany()
to manage memory effectively when dealing with extensive datasets.
- Querying: Utilize methods like
- Installation: Use
By integrating SQLAlchemy into your Python backend projects, you’re equipped with a powerful tool that not only simplifies database interactions but also ensures your application’s data integrity and efficiency.
Whether you’re working on small projects or enterprise-level applications, SQLAlchemy’s flexibility and comprehensive functionality make it an indispensable asset in the Python developer’s toolkit.
8) Celery for Asynchronous Task Queues
Celery is a powerful, distributed task queue framework for Python, offering both flexibility and reliability for processing large amounts of messages.
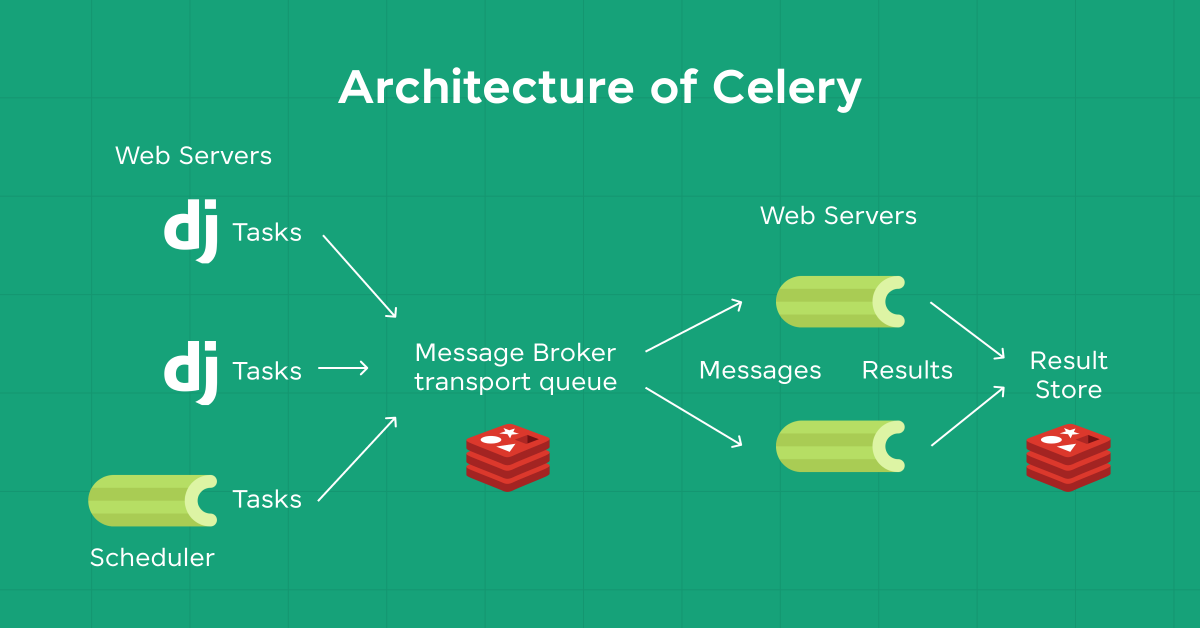
It’s compatible with message brokers like RabbitMQ or Redis, which act as the middleman for sending and receiving messages. Here’s a breakdown of how Celery functions and its key components:
- Task Execution:
- Producer and Consumer Model: Celery can act as both, where the producer sends tasks to the queue, and the consumer processes them.
- Concurrency Models: Utilizes multiprocessing, Eventlet, or Gevent for executing tasks concurrently, enhancing performance.
- Asynchronous and Synchronous Tasks: Supports both execution methods, allowing tasks to be processed either in the background (asynchronously) or the foreground (synchronously).
- Use Cases:
- Background Mailing: Automates sending emails without blocking the main application flow.
- Report Generation: Handles heavy computations for generating reports in the background.
- Logging and Error Reporting: Efficiently manages logging and error reporting tasks, improving application reliability.
- Getting Started with Celery:
- Installation: Requires Python, pip, and a compatible message broker (RabbitMQ/Redis).
- Creating a Celery Instance: Define a Celery instance in your Python project to manage tasks.
- Defining Tasks: Tasks are defined as functions, which can be called using the
.delay()
method for asynchronous execution. - Running a Celery Worker: A worker needs to be started to listen for tasks in the queue and execute them.
- Scheduling with Celery Beat: For periodic tasks, the Celery Beat scheduler allows defining tasks that should run at regular intervals.
Celery’s open-source nature, under the BSD License, and its large, diverse community contribute to its continuous improvement and support.
Its flexibility in task execution and scheduling makes it an indispensable tool for Python backend developers, especially when handling operations that are too time-consuming or complex to run in a synchronous request/response cycle.
9) Redis
Redis, an open-source, in-memory data store, significantly enhances Python backends by offering rapid data retrieval since it stores data directly in memory.
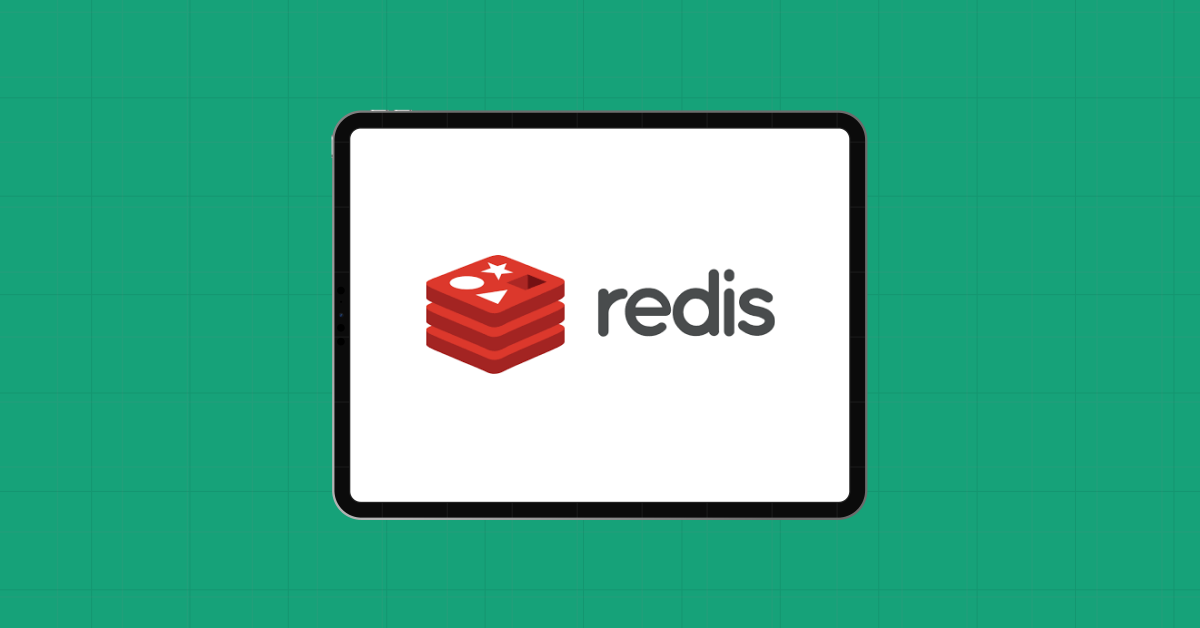
This feature is particularly beneficial for applications requiring high-speed access to data, such as user session management or real-time analytics.
Redis supports a wide range of data structures like strings, lists, sets, hashes, sorted sets, and more, making it versatile for various caching needs.
- Data Persistence and Real-Time Messaging:
- Redis provides data persistence options, ensuring that data is not lost even after a system reboot by storing it on disk.
- Features a Pub/Sub system for real-time messaging and event handling, which is crucial for applications that rely on instant data updates or notifications.
- Caching Benefits:
- Improved Performance: By caching frequently requested data in memory, Redis drastically reduces response times, enhancing the overall speed and efficiency of applications.
- Scalability and Cost Savings: It helps distribute the load on backend systems, making applications more scalable and potentially leading to significant cost savings in server resources and operational expenses.
- Enhanced User Experience: Faster load times and responsiveness, thanks to efficient caching, contribute to a smoother and more enjoyable user experience.
- Redis finds extensive use in caching various types of content, including user sessions, API endpoint responses, articles, images, and user-generated data. Its ability to cache real-time data makes it indispensable for dashboard and analytics applications.
- Major companies like Twitter, Netflix, and Uber leverage Redis caching for storing recent tweets, caching viewing history and preferences, and managing real-time ride requests, respectively.
- However, it’s important to note that while Redis excels in read-heavy workloads and real-time data requirements, it may not be suitable for scenarios involving large data sets, high write intensity, or complex querying.
- Integrating Redis into Python applications is streamlined with the Redis Python library, simplifying the caching process. This involves storing API responses or computed results under corresponding keys for future use, directly serving this data from Redis, and setting appropriate expiration times to ensure data freshness.
- Redis also supports advanced caching strategies like write-behind caching, which can flatten peaks in demand, batch multiple writes, and offload the primary database, further illustrating its flexibility and utility in enhancing Python backends.
Kickstart your Programming journey by enrolling in GUVI’s Python Career Program where you will master technologies like multiple exceptions, classes, OOPS concepts, dictionaries, and many more, and build real-life projects.
Alternatively, if you would like to explore Python through a Self-Paced course, try GUVI’s Python Self-Paced course.
Conclusion
Through the exploration of critical technologies and frameworks vital for excelling as a Python backend developer, our discussion underscores the indispensable role these elements play in crafting robust, efficient, and scalable backend systems.
These tools not only facilitate the development of high-quality applications but also ensure that developers can meet and exceed the increasingly sophisticated expectations of users and clients alike.
As the field continues to advance, staying abreast of these technologies and embracing continuous learning will remain paramount for those seeking to forge a successful career in Python backend development.
Also Explore: Interaction Between Frontend and Backend: Important Process That You Should Know [2024]
FAQs
Which technology is used for backend?
Various technologies are used for backend development, including but not limited to Node.js, Ruby on Rails, Django, Flask, and .NET.
What are the 3 languages used by backend programmers?
Backend programmers commonly use languages such as Python, JavaScript (Node.js), and Ruby.
Is Python a backend language?
Yes, Python is commonly used as a backend language due to its versatility and extensive libraries for web development. Read the article above to learn more.
Did you enjoy this article?