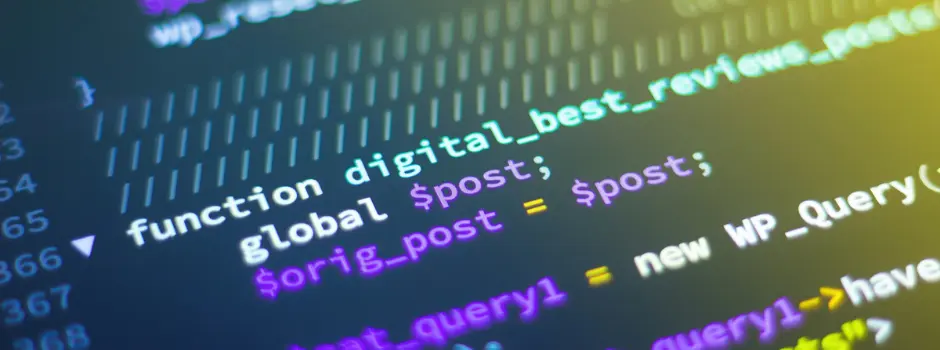
6 Essential Python Modules: A Comprehensive Guide
Mar 27, 2024 4 Min Read 326 Views
(Last Updated)
In the dynamic world of programming, Python stands out with over 137,000 libraries available, offering unparalleled versatility and efficiency.
Exploring Python modules is crucial for leveraging its rich ecosystem to solve various programming challenges, from Machine Learning to Web Development.
Understanding how to navigate and utilize these essential Python modules can significantly enhance your programming projects.
This guide aims to introduce you to 6 fundamental modules in Python, focusing on their functionality, ease of use, and how to seamlessly integrate them into your work for optimized performance.
Table of contents
- 6 Must-Know Python Modules
- 1) os Module: Navigating Filesystem and Environment
- 2) sys Module
- 3) math Module: Mathematical Operations
- 4) datetime Module: Managing Dates and Times
- 5) random Module: Generating Random Data
- 6) json Module: Working with JSON Data
- Conclusion
- FAQs
- What are the most useful built-in modules in Python?
- Which module is best for GUI in Python?
- What is turtle used for in Python?
- What is a class in Python?
6 Must-Know Python Modules
Let us now discuss these important Python modules along with all their functionalities and uses:
1) os Module: Navigating Filesystem and Environment
The os module in Python is a versatile Python toolkit for interacting with your operating system, providing a portable way to use OS-dependent functionality. This includes but is not limited to:
- File and Directory Management: Easily navigate the filesystem, create directories, change the current working directory, and list files and subdirectories using methods like os.getcwd(), os.chdir(), os.mkdir(), and os.listdir().
- Environment and Process Handling: Manage environment variables and process parameters with functions such as os.environ for accessing and modifying environment variables, and os.getpid() for getting the current process ID.
- System-Specific Operations: While some functions, such as os.dup(), os.fchmod(), and os.open() are exclusive to Unix systems, Python’s UTF-8 Mode (introduced in Python 3.7) ensures broader compatibility and performance across different platforms. This mode can be toggled using the -X utf8 command line option or the PYTHONUTF8 environment variable.
It’s crucial to note that directly using os methods instead of executing shell commands is recommended for enhanced performance, portability, and security.
However, be mindful of OS compatibility issues and permission errors, which can be mitigated by consulting the documentation for function support and ensuring the necessary permissions are in place.
Must Read: Top 12 Key Benefits of Learning Python in 2024
2) sys Module
Transitioning from the os module’s exploration of filesystem and environment interactions, we delve into the sys module, a cornerstone for Python developers seeking to fine-tune their scripts and applications with the Python interpreter.
Sys stands out by offering access to variables and functions closely tied to the interpreter itself, facilitating a deeper integration with Python’s runtime environment.
Key functionalities include:
- Command Line Arguments: Through sys.argv, developers can retrieve arguments passed to the script, enhancing script flexibility.
- Interpreter Operations: Functions like sys.exit() allow for clean exits, while sys.version and sys.version_info provide version details critical for compatibility checks.
- Environment Configuration: With sys.path for module search paths, and sys.getdefaultencoding() for managing string encoding, the module offers essential tools for environment setup.
- Recursion and Memory Management: sys.getrecursionlimit() and sys.setrecursionlimit() help manage the depth of recursion, preventing stack overflows, and sys.maxsize reveals the maximum integer size, aiding in memory management.
Its capabilities are pivotal for developers aiming to create robust, efficient Python applications, offering insights into the interpreter and facilitating crucial runtime configurations.
Also Explore: Python Objects 101: How to Create and Master Them With Real-World Projects
3) math Module: Mathematical Operations
With Python’s math module, you’ll discover a powerhouse of mathematical functions essential for executing a wide range of mathematical operations efficiently.
Here’s a breakdown to guide you through its capabilities:
- Core Functions and Constants:
- Number-theoretic Functions: factorial(), gcd(), lcm()
- Representation Functions: ceil(), floor(), trunc()
- Logarithmic and Power Functions: exp(), log(), pow(), sqrt()
- Trigonometric Functions: sin(), cos(), tan(), acos(), asin(), atan()
- Special Functions: gamma(), lgamma(), erf(), erfc()
- Constants: pi, e, tau, inf, nan
It’s important to note that the math module does not support complex numbers. For operations involving complex numbers, the cmath module should be utilized instead.
This distinction ensures that you’re equipped with the right tools for both real and complex mathematical challenges.
The efficiency of the math module stems from its design as a thin wrapper around the C platform’s mathematical functions.
This allows for high-performance computations, making it an invaluable resource for tasks ranging from calculating permutations and combinations to simulating periodic functions and solving quadratic equations.
To leverage these capabilities, simply start by importing the module with import math. This unlocks a suite of functions and constants that are fundamental for mathematical calculations in Python, providing you with a robust toolkit to tackle a variety of programming tasks.
Also Explore: 10 Best Python Libraries for Data Science Career [2024]
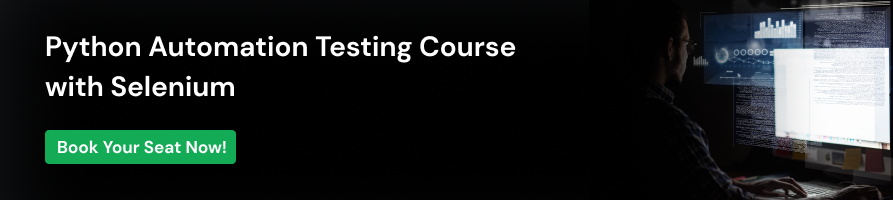
4) datetime Module: Managing Dates and Times
Python’s datetime module is a cornerstone for managing dates and times, providing five main object classes to tackle date and time manipulation with precision. Here’s a closer look at its capabilities:
- Conversion Methods: The strptime() and strftime() methods are invaluable for converting between strings and datetime objects, offering flexibility in handling date and time formats.
- Arithmetic Operations: The module supports comprehensive date and time arithmetic, allowing for operations like addition, subtraction, and comparisons between datetime objects.
- Time Zone and Parsing Support: With the inclusion of the zoneinfo module and compatibility with third-party libraries like dateutil and DateType, managing time zones and parsing dates becomes significantly easier. This is crucial for applications that require accurate time zone handling.
Furthermore, datetime distinguishes between “aware” and “naive” objects, providing a framework for dealing with time zones effectively.
Aware objects contain time zone information, enabling precise comparisons, while naive objects do not, simplifying scenarios where time zone details are unnecessary.
It also offers the timezone class for representing simple time zones with fixed offsets from UTC, enhancing its utility for global applications.
Key constants such as MINYEAR, MAXYEAR, and UTC are exported, alongside immutable object types like date, time, datetime, timedelta, tzinfo, and timezone, ensuring robust and flexible date and time manipulation.
Additionally, efficient pickling of date and time objects via the pickle module is supported, facilitating easy storage and retrieval of datetime objects.
Must Read: 20 Best YouTube Channels to Learn Python in 2024
5) random Module: Generating Random Data
Transitioning smoothly from the precise world of dates and times, we now move towards randomness with Python’s random module.
This one will be your go-to for generating pseudo-random data, crucial for simulations, testing, and gaming. Here’s a breakdown of its key functionalities:
- Basic Random Functions:
- random(): Generates a random float in the range 0.0 <= X < 1.0.
- randint(a, b): Returns a random integer N such that a <= N <= b.
- randrange(start, stop[, step]): Fetches a randomly selected element from range(start, stop, step).
- Advanced Random Functions:
- choices(population, weights=None, k=1): Selects k elements from population with or without replacement, influenced by weights.
- shuffle(x): Randomly shuffles the sequence x in place.
- sample(population, k): Draws a k length list of unique elements from population.
- Statistical Distributions:
- uniform(a, b): Generates a random float N such that a <= N <= b.
- normalvariate(mu, sigma): Returns a random float with the normal distribution.
- expovariate(lambd): Draws a random float with the exponential distribution.
The random module operates on the Mersenne Twister algorithm, ensuring high-quality randomness with a 53-bit precision. Remember, instances of the Random class do not share state, allowing for isolated random generation streams.
Whether you’re simulating dice rolls or selecting random elements from a list, it equips you with a comprehensive toolkit for pseudo-random data generation, enhancing the versatility of your Python projects.
Learn About How To Use Global Variables Inside A Function In Python?
6) json Module: Working with JSON Data
Navigating JSON data in Python becomes straightforward with the json module, a powerful tool for both serialization—converting Python objects into JSON format—and deserialization—the reverse process. Here’s how to harness its capabilities:
- Working with JSON Data:
- Deserialization:
- json.loads(): Parses a JSON string, transforming it into a Python dictionary.
- json.load(): Reads from a file-like object, turning JSON encoded data into Python objects.
- Serialization:
- json.dumps(): Converts a Python object into a JSON formatted string, with options like indent for pretty-printing, separators to define how items are separated, and sort_keys to sort the dictionary keys.
- json.dump(): Writes Python objects as JSON formatted data to a file-like object.
- Deserialization:
- Advanced Techniques:
- Custom encoding and decoding of Python objects can be achieved using the default parameter in dump() and dumps() for encoding, and object_hook in load() and loads() for decoding. This flexibility allows for the handling of complex types beyond the standard JSON data types.
- Practical Applications:
- JSON’s format of nested dictionaries, lists, and scalar values is prevalent in web APIs. Python’s json module simplifies fetching and working with data from these sources, making tasks like consuming a web API or configuring application settings through JSON files both efficient and straightforward.
Its integration into Python supports a wide range of applications, from web development to data analysis, by facilitating easy exchange and manipulation of data in the popular JSON format.
Also Read: Build your own personal voice assistant like Siri, Alexa using Python
Conclusion
Through this comprehensive guide, we have gone through key modules that stand at the core of Python’s utility and versatility for developers across fields.
The significance of understanding these modules cannot be overstated, as it empowers developers to harness the full potential of Python’s extensive libraries for optimized performance and innovative solutions.
As the programming challenges evolve and become more complex, the knowledge of these essential Python modules and their adept utilization will remain indispensable.
For those who seek to deepen their programming expertise and broaden the scope of their projects, learning Full Stack Development will be a great investment and the stepping stone to a successful career in tech.
Must Explore: Learn Python in 2024: 10 Interesting Reasons to Do So!
FAQs
What are the most useful built-in modules in Python?
Some of the most useful built-in modules in Python include os, sys, math, random, and datetime.
Which module is best for GUI in Python?
Tkinter is often considered the best module for GUI programming in Python due to its simplicity and ease of use.
What is turtle used for in Python?
The turtle module in Python is primarily used for creating simple graphics and drawings.
Did you enjoy this article?